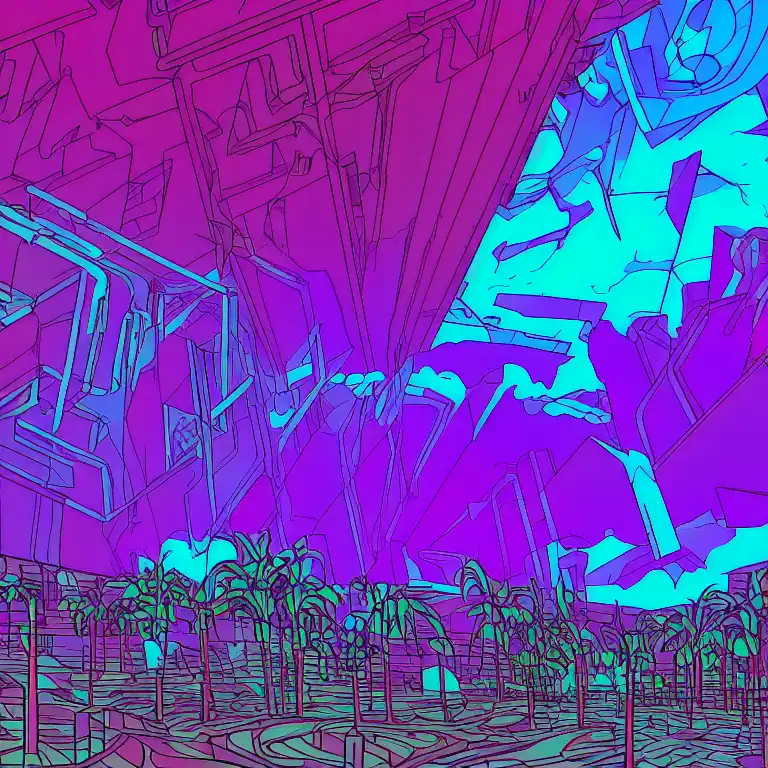
June 6th, 2023
Welcome back to our Go programming series! In our previous post, we covered Gin routing and middleware, two powerful features that allow you to build complex web applications with ease. Now, let's dive into databases and show you how to connect your Go web application to a PostgreSQL database using the popular pgx
library.
PostgreSQL and pgx
PostgreSQL is a popular open-source relational database management system that provides robust features and scalability. pgx
is a PostgreSQL driver and toolkit for Go that provides a fast and efficient way to interact with PostgreSQL databases.
To use pgx
, you need to install the library using the following command:
go get github.com/jackc/pgx/v4
Connecting to a PostgreSQL Database
Here's an example of using pgx
to connect to a PostgreSQL database and retrieve data:
package main import ( "log" "github.com/jackc/pgx/v4" ) func main() { // define the PostgreSQL connection string connString := "postgres://user:password@localhost:5432/mydb" // create a new PostgreSQL connection conn, err := pgx.Connect(context.Background(), connString) if err != nil { log.Fatal(err) } defer conn.Close() // execute a SQL query rows, err := conn.Query(context.Background(), "SELECT * FROM users") if err != nil { log.Fatal(err) } defer rows.Close() // iterate over the query results and print them to the console for rows.Next() { var id int var name string err := rows.Scan(&id, &name) if err != nil { log.Fatal(err) } log.Printf("ID: %d, Name: %s\n", id, name) } if err := rows.Err(); err != nil { log.Fatal(err) } }
In this example, we define a PostgreSQL connection string that specifies the username, password, host, port, and database name.
We create a new PostgreSQL connection using the pgx.Connect
method, passing in the connection string. We use the defer
statement to ensure that the connection is closed when the function returns.
We execute a SQL query using the conn.Query
method, passing in the SQL query string. We use the defer
statement to ensure that the query results are closed when the function returns.
We iterate over the query results using the rows.Next
method and print them to the console using the log.Printf
function.
Conclusion
In this post, we covered how to connect to a PostgreSQL database using the pgx
library. In the next post, we'll show you how to integrate pgx
into your Gin web application and build a simple CRUD API. Stay tuned!