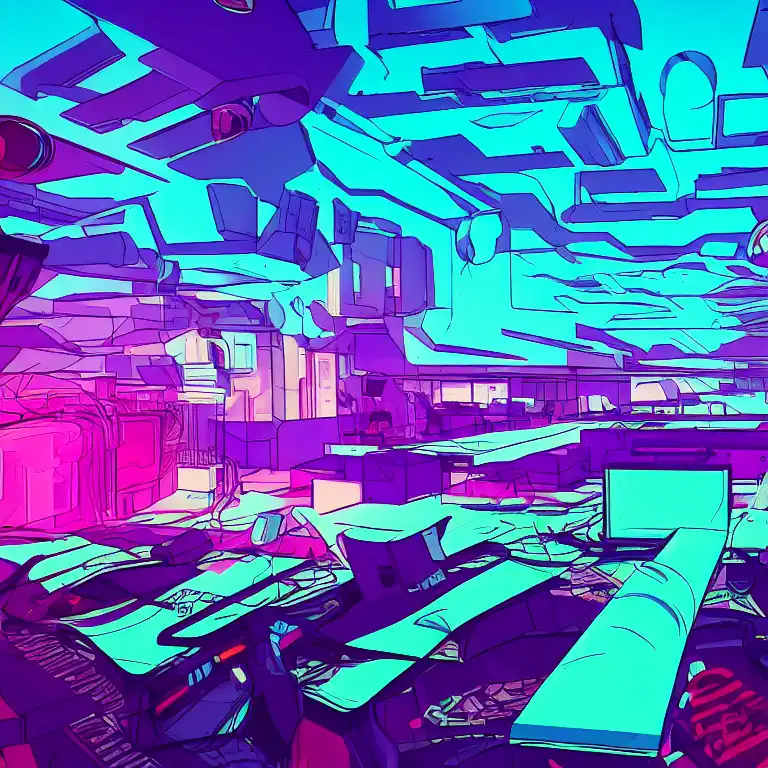
May 19th, 2023
In the previous post, we covered MongoDB's document model and showed you how to work with data in MongoDB. In this post, we'll introduce MongoDB's aggregation framework, which allows you to perform complex data analysis operations on your MongoDB data.
Aggregation Pipeline
The aggregation framework in MongoDB uses a pipeline architecture to process data. The pipeline consists of stages, where each stage performs a specific operation on the data. The output of one stage becomes the input for the next stage in the pipeline.
The aggregation pipeline can be divided into three parts: input stage, processing stage, and output stage. The input stage is where you specify the input data for the pipeline. The processing stage is where you perform the data analysis operations, and the output stage is where you define the output format for the pipeline.
Aggregation Operators
MongoDB's aggregation framework provides a wide range of operators for data analysis operations. Here are some of the most commonly used operators:
$match
: Filters the documents in the input data based on a specified condition.$project
: Reshapes the documents in the input data by including, excluding, or renaming fields.$group
: Groups the documents in the input data based on a specified key and performs aggregation operations on each group.$sort
: Sorts the documents in the input data based on a specified field.$limit
: Limits the number of documents in the output data.$skip
: Skips a specified number of documents in the input data.$lookup
: Performs a left outer join between two collections based on a specified condition.
Example Aggregation Pipeline
Let's walk through an example aggregation pipeline to illustrate how the pipeline works. Suppose we have a collection called orders
that contains documents with the following fields:
{ _id: ObjectId("615c9fb63fcb8607b91a12a4"), customer: "John", product: "TV", price: 500, date: ISODate("2021-10-06T00:00:00Z") }
Here's an example aggregation pipeline that calculates the total revenue by customer:
db.orders.aggregate([ { $group: { _id: "$customer", total: { $sum: "$price" } } }, { $sort: { total: -1 } } ])
This pipeline has two stages: $group
and $sort
. The $group
stage groups the documents by the customer
field and calculates the sum of the price field for each group. The output of the $group
stage is a document with two fields: _id
(the grouping key) and total
(the sum of the price field for the group). The $sort
stage sorts the output data in descending order based on the total
field.
Conclusion
In this post, we introduced MongoDB's aggregation framework, which allows you to perform complex data analysis operations on your MongoDB data. We walked through the aggregation pipeline and the most commonly used aggregation operators. We also provided an example aggregation pipeline to illustrate how the pipeline works. In the next post, we'll cover MongoDB's indexing capabilities, which allow you to optimize your queries for performance. Stay tuned!