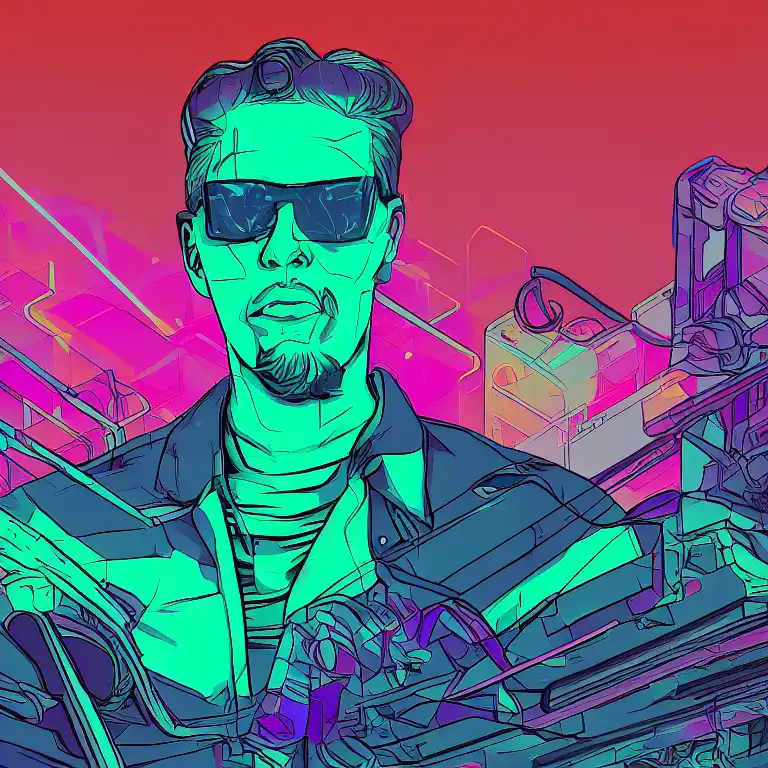
May 20th, 2023
In the previous post, we introduced MongoDB's aggregation framework, which allows you to perform complex data analysis operations on your MongoDB data. In this post, we'll cover MongoDB's indexing capabilities, which allow you to optimize your queries for performance.
Indexing Basics
An index in MongoDB is similar to an index in a book. It provides a quick way to look up information based on a specific field or set of fields. An index is stored separately from the data, so it doesn't affect the storage requirements of the database.
MongoDB supports a wide range of index types, including single-field indexes, compound indexes, geospatial indexes, text indexes, and more. You can create indexes using the createIndex()
method, which takes a document that specifies the index keys and options.
Indexing Best Practices
Here are some best practices for indexing in MongoDB:
- Index the fields that you frequently query on.
- Use compound indexes for queries that involve multiple fields.
- Use sparse indexes for fields with a low cardinality (i.e. fields with many null or missing values).
- Consider using TTL indexes for documents with a time-to-live (TTL) property.
- Regularly monitor your indexes and remove unused indexes to improve performance.
Example Index Creation
Let's walk through an example of creating an index in MongoDB. Suppose we have a collection called orders
that contains documents with the following fields:
{ _id: ObjectId("615c9fb63fcb8607b91a12a4"), customer: "John", product: "TV", price: 500, date: ISODate("2021-10-06T00:00:00Z") }
To create an index on the customer
field, we can run the following command:
db.orders.createIndex({ customer: 1 })
This creates a single-field index on the customer
field in ascending order.
Query Optimization
Indexes can significantly improve query performance in MongoDB. When a query is executed, MongoDB checks if an index exists for the fields in the query. If an index exists, MongoDB uses the index to quickly locate the matching documents. If an index does not exist, MongoDB must perform a full collection scan, which can be slow for large collections.
Conclusion
In this post, we covered MongoDB's indexing capabilities, which allow you to optimize your queries for performance. We walked through the basics of indexing, indexing best practices, and provided an example of index creation. We also discussed how indexes can improve query performance in MongoDB. In the next post, we'll cover MongoDB's security features, which allow you to protect your data from unauthorized access. Stay tuned!