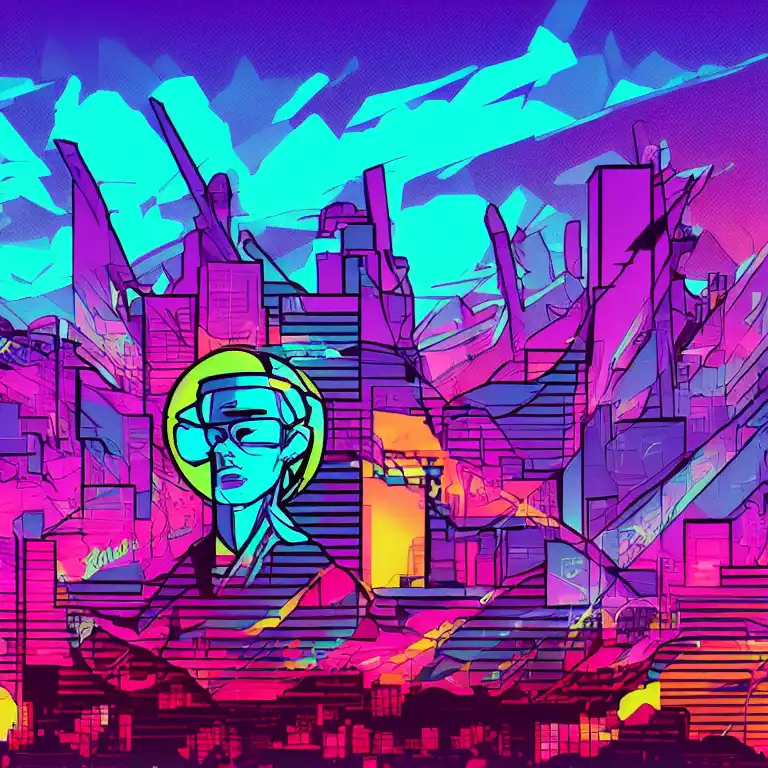
May 18th, 2023
In the previous post, we walked you through the process of setting up a MongoDB environment on a Linux system. In this post, we'll dive deeper into MongoDB's document model and show you how to work with data in MongoDB.
MongoDB's Document Model
MongoDB is a document-oriented database, which means that data is stored in documents rather than tables. A document is a set of key-value pairs that represent a single unit of data. Documents in MongoDB are stored in collections, which are similar to tables in relational databases.
MongoDB documents are stored in a format called BSON (Binary JSON), which is a binary-encoded representation of JSON data. BSON allows for efficient storage and retrieval of data in MongoDB.
Working with Data in MongoDB
Now that you understand MongoDB's document model, let's walk through some common operations for working with data in MongoDB.
Inserting Data
To insert data into a collection, you can use the insertOne()
or insertMany()
methods. For example, to insert a single document into a collection, you can run the following command:
db.mycollection.insertOne({ name: "Jane", age: 25 })
To insert multiple documents into a collection, you can use the insertMany()
method:
db.mycollection.insertMany([ { name: "Alice", age: 32 }, { name: "Bob", age: 28 }, { name: "Charlie", age: 40 } ])
Querying Data
To retrieve data from a collection, you can use the find()
method. The find()
method returns a cursor object, which you can use to iterate over the matching documents. For example, to retrieve all documents from a collection, you can run the following command:
db.mycollection.find()
To retrieve a specific document based on a query, you can pass a query object to the find()
method. For example, to retrieve all documents where the age
field is greater than 30, you can run the following command:
db.mycollection.find({ age: { $gt: 30 } })
Updating Data
To update a document in a collection, you can use the updateOne()
or updateMany()
methods. For example, to update the age
field of a document with a specific name, you can run the following command:
db.mycollection.updateOne({ name: "Alice" }, { $set: { age: 35 } })
Deleting Data
To delete a document from a collection, you can use the deleteOne()
or deleteMany()
methods. For example, to delete a document with a specific name, you can run the following command:
db.mycollection.deleteOne({ name: "Bob" })
Conclusion
In this post, we've covered MongoDB's document model and shown you how to work with data in MongoDB. We've walked through common operations for inserting, querying, updating, and deleting data in MongoDB. In the next post, we'll cover MongoDB's aggregation framework, which allows you to perform complex data analysis operations. Stay tuned!