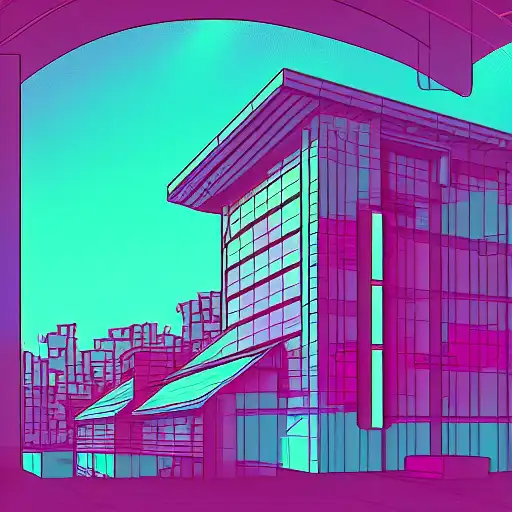
May 9th, 2023
Angular services are a way to organize and share code between components in your application. Services provide a way to keep your code modular and maintainable by separating concerns and promoting reusability. In this tutorial, we'll explore how to use Angular services to organize and share code between components.
Creating a Service
To create a service in Angular, we can use the "ng generate service" command or create a new TypeScript file manually. Let's create a new service called "UserService" that provides a simple "getUsers" method:
ng generate service user
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class UserService { getUsers() { return [ { id: 1, name: 'John Doe', email: 'johndoe@example.com' }, { id: 2, name: 'Jane Smith', email: 'janesmith@example.com' }, ]; } }
In this example, we're using the "Injectable" decorator to mark the class as a service. We're also using the "providedIn" property to specify that the service should be provided at the root level. Finally, we're defining a simple "getUsers" method that returns an array of user objects.
Using a Service in a Component
Now that we have our "UserService" service, let's use it in a component. Let's create a new component called "UserListComponent" that displays a list of users:
ng generate component user-list
import { Component, OnInit } from '@angular/core'; import { UserService } from '../user.service'; @Component({ selector: 'app-user-list', template: ` <h1>User List</h1> <ul> <li *ngFor="let user of users">{{ user.name }} - {{ user.email }}</li> </ul> `, }) export class UserListComponent implements OnInit { users = []; constructor(private userService: UserService) {} ngOnInit() { this.users = this.userService.getUsers(); } }
In this example, we're using the "UserService" service in the "UserListComponent" component by injecting it into the constructor. We're also using the "ngOnInit" lifecycle hook to call the "getUsers" method of the service and store the result in the "users" property.
Conclusion
In this tutorial, we've explored how to use Angular services to organize and share code between components. By using services, we can keep our code modular and maintainable by separating concerns and promoting reusability. In the next tutorial, we'll explore how to use Angular's routing module to navigate between components in your application.