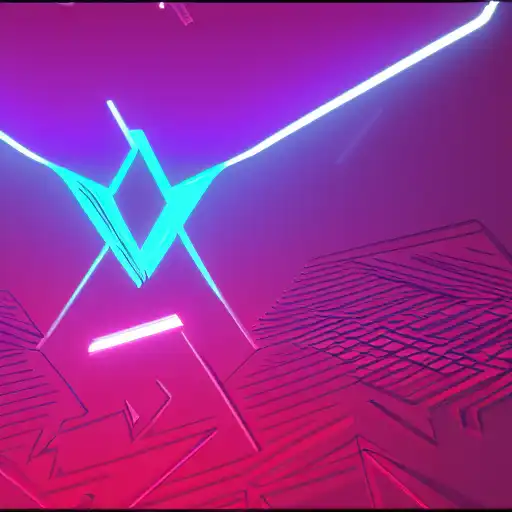
May 10th, 2023
Angular's routing module provides a powerful way to navigate between components in your application. By using the "RouterModule" and "Routes" classes, we can define routes and their corresponding components, and Angular will handle the navigation between them. In this tutorial, we'll explore how to use Angular's routing module to navigate between components in your application.
Creating a Route
To create a route in Angular, we can use the "RouterModule" and "Routes" classes to define the routes and their corresponding components. Let's create a new route that displays the "UserListComponent" component:
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { UserListComponent } from './user-list/user-list.component'; const routes: Routes = [ { path: 'users', component: UserListComponent }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule], }) export class AppRoutingModule {}
In this example, we're using the "Routes" class to define a route with a path of "users" and a corresponding component of "UserListComponent". We're also using the "RouterModule.forRoot" method to configure the routes and the "AppRoutingModule" class to export the routing module.
Using a Route in a Component
Now that we have our "AppRoutingModule" set up, let's use the route in a component. Let's update the "AppComponent" component to display a link to the "UserListComponent" component:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>My App</h1> <nav> <a routerLink="/users">Users</a> </nav> <router-outlet></router-outlet> `, }) export class AppComponent {}
In this example, we're using the "routerLink" directive to navigate to the "UserListComponent" component when the link is clicked. We're also using the "router-outlet" directive to display the corresponding component for the current route.
Navigating Programmatically
In addition to using links to navigate between components, we can also navigate programmatically using the "Router" service. Let's update the "UserListComponent" to navigate to a new route when a user is selected:
import { Component, OnInit } from '@angular/core'; import { Router } from '@angular/router'; import { UserService } from '../user.service'; @Component({ selector: 'app-user-list', template: ` <h1>User List</h1> <ul> <li *ngFor="let user of users" (click)="onSelect(user)">{{ user.name }} - {{ user.email }}</li> </ul> `, }) export class UserListComponent implements OnInit { users = []; constructor(private userService: UserService, private router: Router) {} ngOnInit() { this.users = this.userService.getUsers(); } onSelect(user) { this.router.navigate(['/users', user.id]); } }
In this example, we're using the "Router" service to navigate to a new route when a user is selected. We're also using the "click" event to call the "onSelect" method when a user is clicked.
Conclusion
In this tutorial, we've explored how to use Angular's routing module to navigate between components in your application. By using the "RouterModule" and "Routes" classes, we can define routes and their corresponding components, and Angular will handle the navigation between them. In the next and final tutorial of this series, we'll wrap up everything we learned.