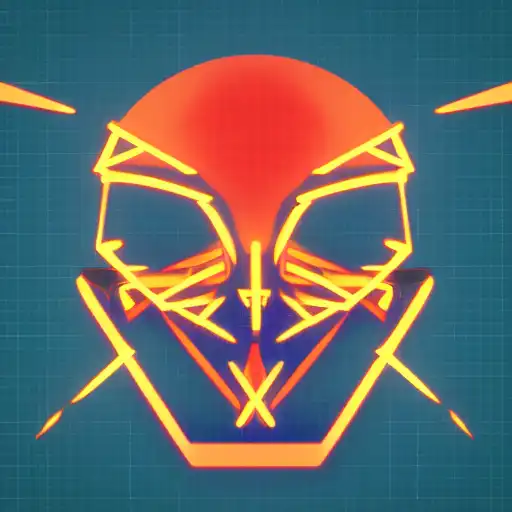
May 8th, 2023
Submitting data from a form to a server is a common task in web applications, and Angular provides a powerful HTTP module to make this task easy. In this tutorial, we'll explore how to use Angular's HTTP module to submit data from a form to a server.
Submitting Form Data with HTTP
To submit form data with Angular's HTTP module, we need to import the "HttpClient" module and use the "post" method to make a POST request to the server. Let's update the "FormComponent" from the previous tutorial to submit the form data:
import { Component } from '@angular/core'; import { FormBuilder, Validators } from '@angular/forms'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-form', template: ` <h1>Submit Form</h1> <form [formGroup]="form" (ngSubmit)="onSubmit()"> <label> Name: <input type="text" formControlName="name" /> </label> <div *ngIf="name.invalid && (name.dirty || name.touched)"> <div *ngIf="name.errors.required">Name is required</div> </div> <br /> <label> Email: <input type="email" formControlName="email" /> </label> <div *ngIf="email.invalid && (email.dirty || email.touched)"> <div *ngIf="email.errors.required">Email is required</div> <div *ngIf="email.errors.email">Email is invalid</div> </div> <br /> <button type="submit" [disabled]="form.invalid">Submit</button> </form> `, }) export class FormComponent { form = this.fb.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]], }); get name() { return this.form.get('name'); } get email() { return this.form.get('email'); } constructor(private fb: FormBuilder, private http: HttpClient) {} onSubmit() { const url = 'http://localhost:3000/submit'; const data = this.form.value; this.http.post(url, data).subscribe((response) => { console.log(response); }); } }
In this example, we're using the "HttpClient" module to make a POST request to the server. We're also using the "post" method to submit the form data to the server.
Using the Server to Handle Form Submissions
Now that we can submit form data with the "FormComponent", let's set up the server to handle form submissions. We'll use the same "json-server" package that we used in a previous tutorial to set up a server that handles form submissions.
First, let's create a simple JSON file called "db.json" that contains an empty array:
{ "data": [] }
Next, let's start the server and serve the JSON file:
json-server --watch db.json
Finally, let's update the "FormComponent" to submit the form data to the server:
import { Component } from '@angular/core'; import { FormBuilder, Validators } from '@angular/forms'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-form', template: ` <h1>Submit Form</h1> <form [formGroup]="form" (ngSubmit)="onSubmit()"> <label> Name: <input type="text" formControlName="name" /> </label> <div *ngIf="name.invalid && (name.dirty || name.touched)"> <div *ngIf="name.errors.required">Name is required</div> </div> <br /> <label> Email: <input type="email" formControlName="email" /> </label> <div *ngIf="email.invalid && (email.dirty || email.touched)"> <div *ngIf="email.errors.required">Email is required</div> <div *ngIf="email.errors.email">Email is invalid</div> </div> <br /> <button type="submit" [disabled]="form.invalid">Submit</button> </form>`, }) export class FormComponent { form = this.fb.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]], }); get name() { return this.form.get('name'); } get email() { return this.form.get('email'); } constructor(private fb: FormBuilder, private http: HttpClient) {} onSubmit() { const url = 'http://localhost:3000/data'; const data = this.form.value; this.http.post(url, data).subscribe((response) => { console.log(response); this.form.reset(); }); } }
In this example, we're using the "json-server" package to set up a server that handles form submissions. We're also using the "post" method to submit the form data to the server.
Conclusion
In this tutorial, we've explored how to use Angular's HTTP module to submit data from a form to a server. By using the "HttpClient" module and the "post" method, we can submit form data to a server and handle the response. Angular's HTTP module provides a powerful and flexible way to submit data to a server in your application. In the next tutorial, we'll explore how to use Angular's services to organize and share code between components.