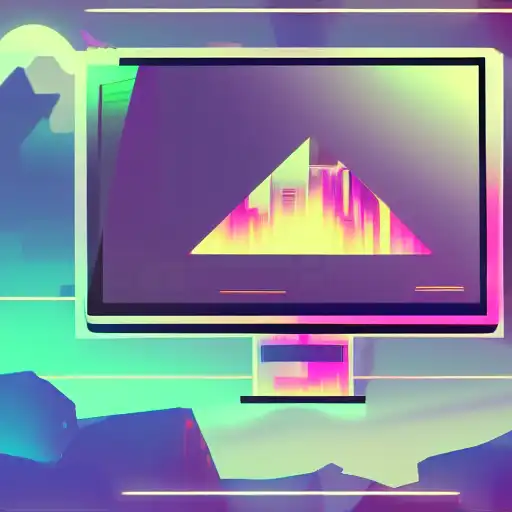
May 7th, 2023
Forms are a critical part of most web applications, and Angular provides a powerful forms module to make creating and validating forms easy. In this tutorial, we'll explore how to use Angular's forms module to create forms in your application.
Creating a Simple Form
To create a form in Angular, we can use the "FormsModule" or "ReactiveFormsModule" module. Let's start by creating a simple form that allows the user to enter their name and email address using the "FormsModule":
import { Component } from '@angular/core'; @Component({ selector: 'app-form', template: ` <h1>Simple Form</h1> <form (ngSubmit)="onSubmit()"> <label> Name: <input type="text" [(ngModel)]="name" name="name" required /> </label> <br /> <label> Email: <input type="email" [(ngModel)]="email" name="email" required /> </label> <br /> <button type="submit">Submit</button> </form> `, }) export class FormComponent { name = ''; email = ''; onSubmit() { console.log(`Name: ${this.name}, Email: ${this.email}`); } }
In this example, we're using the "ngModel" directive to bind the input values to the "name" and "email" properties of the component. We're also using the "ngSubmit" directive to handle form submission.
Adding Validation
Now that we have our simple form set up, let's add validation to the form. We can use Angular's built-in validators or create custom validators to validate the form.
Let's add required validation to the "name" and "email" fields:
import { Component } from '@angular/core'; import { FormBuilder, Validators } from '@angular/forms'; @Component({ selector: 'app-form', template: ` <h1>Validated Form</h1> <form [formGroup]="form" (ngSubmit)="onSubmit()"> <label> Name: <input type="text" formControlName="name" /> </label> <div *ngIf="name.invalid && (name.dirty || name.touched)"> <div *ngIf="name.errors.required">Name is required</div> </div> <br /> <label> Email: <input type="email" formControlName="email" /> </label> <div *ngIf="email.invalid && (email.dirty || email.touched)"> <div *ngIf="email.errors.required">Email is required</div> <div *ngIf="email.errors.email">Email is invalid</div> </div> <br /> <button type="submit" [disabled]="form.invalid">Submit</button> </form> `, }) export class FormComponent { form = this.fb.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]], }); get name() { return this.form.get('name'); } get email() { return this.form.get('email'); } constructor(private fb: FormBuilder) {} onSubmit() { console.log(this.form.value); } }
In this example, we're using the "FormBuilder" to create a form with the "name" and "email" fields. We're also using the "Validators" class to add required and email validation to the fields. We're also using the "formControlName" directive to bind the inputs to the corresponding form controls. We're using the "ngIf" directive to display validation messages when the fields are invalid and have been touched or are dirty.
We're also using the "form.invalid" property to disable the submit button when the form is invalid.
Conclusion
In this tutorial, we've explored how to use Angular's forms module to create and validate forms in your application. By using the "ngModel" and "ngSubmit" directives, we can create simple forms that capture user input. By using the "FormBuilder" and "Validators" class, we can add validation to the form and display validation messages. Angular's forms module provides a powerful and flexible way to create and validate forms in your application. In the next tutorial, we'll explore how to use Angular's HTTP module to submit data from a form to a server.