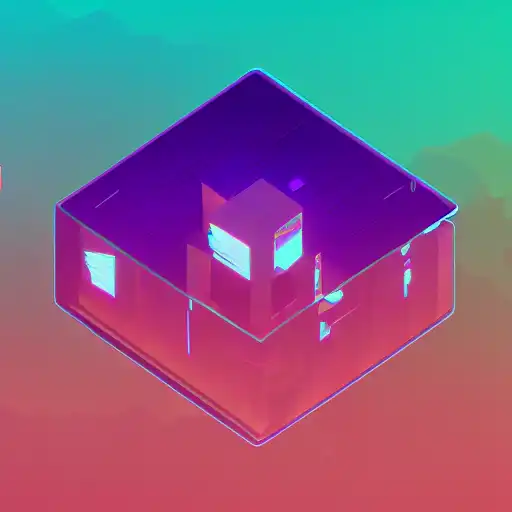
May 6th, 2023
Navigation menus are an essential part of most web applications, and Angular provides a powerful router module to make creating navigation menus easy. In this tutorial, we'll explore how to use Angular's router module to create a navigation menu.
Creating the Navigation Menu
To create a navigation menu in Angular, we need to define the routes for each component and create a template for the menu. Let's start by defining the routes for our components in the "app-routing.module.ts" file:
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home.component'; import { DataComponent } from './data.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'data', component: DataComponent }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule], }) export class AppRoutingModule {}
In this example, we're defining two routes: one for the "HomeComponent" and one for the "DataComponent". We're also using the "forRoot" method to set up the router module and the "exports" property to make the router module available to other modules.
Next, let's create a template for the navigation menu in the "app.component.html" file:
<nav> <a routerLink="/">Home</a> <a routerLink="/data">Data</a> </nav> <router-outlet></router-outlet>
In this example, we're using the "routerLink" directive to create links to the "HomeComponent" and the "DataComponent". We're also using the "router-outlet" directive to display the component that corresponds to the current route.
Using the Navigation Menu
Now that we have our navigation menu set up, let's use it in our application. We'll create a simple "HomeComponent" that displays a message and a "DataComponent" that retrieves data and displays it in a list.
import { Component } from '@angular/core'; @Component({ selector: 'app-home', template: `<h1>Welcome to my app!</h1>`, }) export class HomeComponent {}
import { Component, OnInit } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-data', template: ` <h1>Data</h1> <ul> <li *ngFor="let item of data">{{ item.name }}</li> </ul> `, }) export class DataComponent implements OnInit { data: any[] = []; constructor(private dataService: DataService) {} ngOnInit() { this.dataService.getData().subscribe((response) => { this.data = response.data; }); } }
In this example, we're using the "HomeComponent" to display a welcome message and the "DataComponent" to retrieve and display data.
Conclusion
In this tutorial, we've explored how to use Angular's router module to create a navigation menu. By defining routes for each component and using the "routerLink" and "router-outlet" directives, we can create a navigation menu and display components based on the current route. In the next tutorial, we'll explore how to use Angular's forms module to create forms in our application.