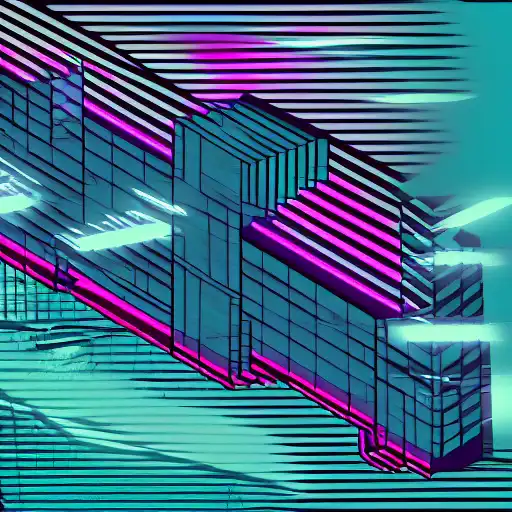
May 5th, 2023
Retrieving data from a server is a common task in web applications, and Angular provides a powerful HTTP module to make this task easy. In this tutorial, we'll explore how to use Angular's HTTP module to retrieve data from a server.
Setting up the Server
Before we can retrieve data from a server, we need to set up a server that serves the data. For this tutorial, we'll use the "json-server" package to set up a simple server that serves a JSON file.
First, let's install the "json-server" package:
npm install -g json-server
Next, let's create a simple JSON file called "data.json" that contains some data:
{ "data": [ { "id": 1, "name": "Item 1" }, { "id": 2, "name": "Item 2" }, { "id": 3, "name": "Item 3" } ] }
Finally, let's start the server and serve the JSON file:
json-server --watch data.json
Now that we have our server set up, let's use Angular's HTTP module to retrieve the data.
Retrieving Data with HTTP
To retrieve data with Angular's HTTP module, we need to import the "HttpClient" module and use the "get" method to make a GET request to the server. Let's create a simple "DataService" that retrieves the data:
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root', }) export class DataService { private url = 'http://localhost:3000/data'; constructor(private http: HttpClient) {} getData(): Observable<any> { return this.http.get<any>(this.url); } }
In this example, we're using the "HttpClient" module to make a GET request to the server. We're also using the "Observable" type to handle the asynchronous nature of HTTP requests.
Using the Service in a Component
Now that we can retrieve data with the "DataService", let's use it in a component. We'll create a simple "DataComponent" that retrieves the data and displays it in a list:
import { Component, OnInit } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-data', template: ` <ul> <li *ngFor="let item of data">{{ item.name }}</li> </ul> `, }) export class DataComponent implements OnInit { data: any[] = []; constructor(private dataService: DataService) {} ngOnInit() { this.dataService.getData().subscribe((response) => { this.data = response.data; }); } }
In this example, we're using the "DataService" in the "DataComponent" to retrieve the data from the server. We're also using the "ngFor" directive to loop over the data and display it in a list.
Conclusion
In this tutorial, we've explored how to use Angular's HTTP module to retrieve data from a server. By importing the "HttpClient" module and using the "get" method, we can make HTTP requests and retrieve data from a server. By creating a service that retrieves the data and using it in a component, we can display the data in our templates. In the next tutorial, we'll explore how to use Angular's router module to create a navigation menu.