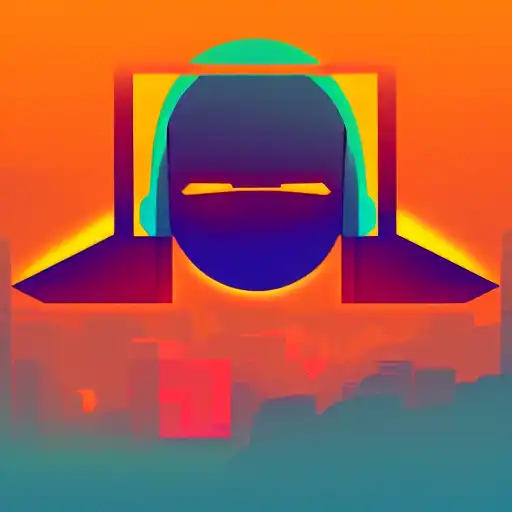
May 4th, 2023
Pipes are a useful feature of Angular that allow us to format and transform data in our templates. In this tutorial, we'll explore how to use pipes to format data in Angular.
Creating a Pipe
To create a pipe in Angular, we can use the "ng generate pipe" command or create a new file manually. Let's create a simple pipe called "ReversePipe" that reverses a string:
import { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'reverse', }) export class ReversePipe implements PipeTransform { transform(value: string): string { return value.split('').reverse().join(''); } }
In this example, we're using the "Pipe" decorator to create a pipe called "ReversePipe". The pipe implements the "PipeTransform" interface, which requires the "transform" method.
The "transform" method takes a value (in this case, a string) and returns the transformed value (in this case, the reversed string).
Using a Pipe in a Component
Now that we have our pipe set up, let's use it in a component. We'll create a simple "ReverseComponent" that takes a string and displays the reversed string using the "ReversePipe":
import { Component } from '@angular/core'; @Component({ selector: 'app-reverse', template: ` <p>{{ message }}</p> <p>Reversed: {{ message | reverse }}</p> `, }) export class ReverseComponent { message = 'Hello, world!'; }
In this example, we're using the "ReversePipe" in the "ReverseComponent" to reverse the "message" string. We're also using the pipe in the template by using the pipe character (|) followed by the pipe name ("reverse").
Conclusion
In this tutorial, we've explored how to use pipes to format data in Angular. Pipes are an essential part of most web applications, and Angular provides a powerful and flexible way to create and use pipes using the "Pipe" decorator and the "PipeTransform" interface. By creating a pipe that transforms data and using it in a component, we can format and transform data in our templates. In the next tutorial, we'll explore how to use Angular's built-in HTTP module to retrieve data from a server.