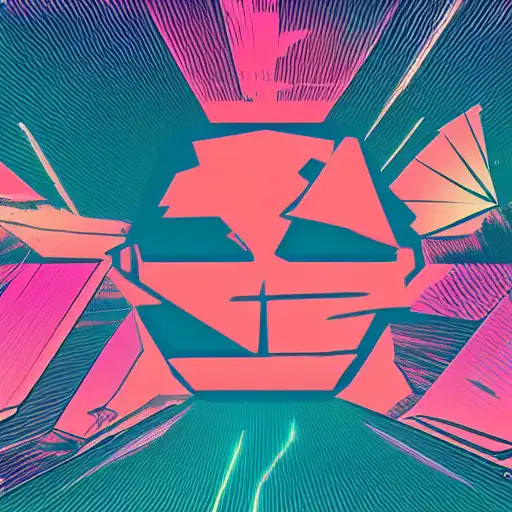
May 3rd, 2023
Directives are a powerful feature of Angular that allow us to manipulate the DOM and add functionality to our templates. In this tutorial, we'll explore how to use directives to manipulate the DOM in Angular.
Creating a Directive
To create a directive in Angular, we can use the "ng generate directive" command or create a new file manually. Let's create a simple directive called "HighlightDirective" that highlights an element when the user hovers over it:
import { Directive, ElementRef, HostListener } from '@angular/core'; @Directive({ selector: '[appHighlight]', }) export class HighlightDirective { constructor(private el: ElementRef) {} @HostListener('mouseenter') onMouseEnter() { this.highlight('yellow'); } @HostListener('mouseleave') onMouseLeave() { this.highlight(null); } private highlight(color: string | null) { this.el.nativeElement.style.backgroundColor = color; } }
In this example, we're using the "Directive" decorator to create a directive called "HighlightDirective". The directive contains a constructor that takes an "ElementRef" parameter, which provides access to the element that the directive is attached to.
We're also using the "HostListener" decorator to listen for the "mouseenter" and "mouseleave" events on the element. When the user hovers over the element, we're using the "highlight" method to set the background color of the element to "yellow". When the user leaves the element, we're setting the background color back to null.
Using a Directive in a Component
Now that we have our directive set up, let's use it in a component. We'll create a simple "HighlightComponent" that displays a div and applies the "HighlightDirective" to it:
import { Component } from '@angular/core'; @Component({ selector: 'app-highlight', template: ` <div appHighlight>Hover over me to highlight</div> `, }) export class HighlightComponent {}
In this example, we're using the "HighlightDirective" in the "HighlightComponent" to apply the highlight effect to the div. We're also using the "appHighlight" selector to apply the directive to the div.
Conclusion
In this tutorial, we've explored how to use directives to manipulate the DOM in Angular. Directives are an essential part of most web applications, and Angular provides a powerful and flexible way to create and use directives using the "Directive" decorator and the "ElementRef" and "HostListener" parameters. By creating a directive that adds functionality to an element and using it in a component, we can manipulate the DOM and add functionality to our templates. In the next tutorial, we'll explore how to use Angular's built-in pipes to format data.