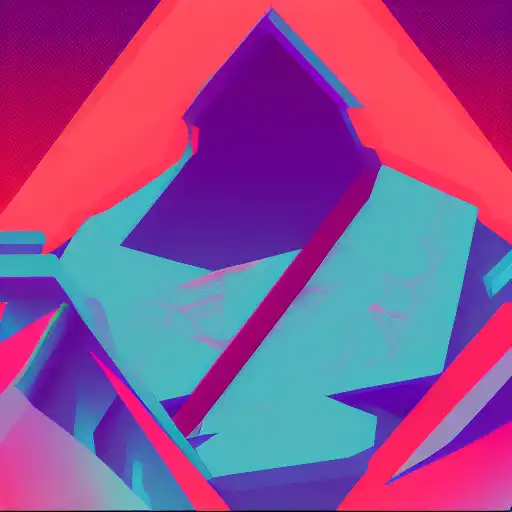
May 2nd, 2023
Services are a key part of Angular and are used to provide functionality that can be shared across components. In this tutorial, we'll explore how to use services to share data between components in Angular.
Creating a Service
To create a service in Angular, we can use the "ng generate service" command or create a new file manually. Let's create a simple service called "DataService" that will store and retrieve data:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class DataService { data: string[] = []; addData(newData: string) { this.data.push(newData); } getData() { return this.data; } }
In this example, we're using the "Injectable" decorator to create a service called "DataService". The service contains a property called "data" that stores an array of strings and two methods: "addData" and "getData".
Using a Service in a Component
Now that we have our service set up, let's use it in a component. We'll create a simple "AddDataComponent" that allows the user to input data and add it to the "DataService":
import { Component } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-add-data', template: ` <input type="text" [(ngModel)]="newData" /> <button (click)="onAdd()">Add Data</button> `, }) export class AddDataComponent { newData = ''; constructor(private dataService: DataService) {} onAdd() { this.dataService.addData(this.newData); this.newData = ''; } }
In this example, we're using the "DataService" in the "AddDataComponent" to add data to the "data" property of the service. We're also using the "ngModel" directive to bind the input field to the "newData" property of the component.
Displaying Data in a Component
Now that we can add data to the "DataService", let's create a component that displays the data. We'll create a simple "DataComponent" that retrieves the data from the "DataService" and displays it in a list:
import { Component } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-data', template: ` <ul> <li *ngFor="let item of data">{{ item }}</li> </ul> `, }) export class DataComponent { data: string[] = []; constructor(private dataService: DataService) {} ngOnInit() { this.data = this.dataService.getData(); } }
In this example, we're using the "DataService" in the "DataComponent" to retrieve the data from the "data" property of the service. We're also using the "ngFor" directive to loop over the data and display it in a list.
Conclusion
In this tutorial, we've explored how to use services to share data between components in Angular. Services are an essential part of most web applications, and Angular provides a powerful and flexible way to create and use services using the "Injectable" decorator. By creating a service that stores and retrieves data and using it in multiple components, we can share data and functionality across our application. In the next tutorial, we'll explore how to use Angular's built-in directives to manipulate the DOM.