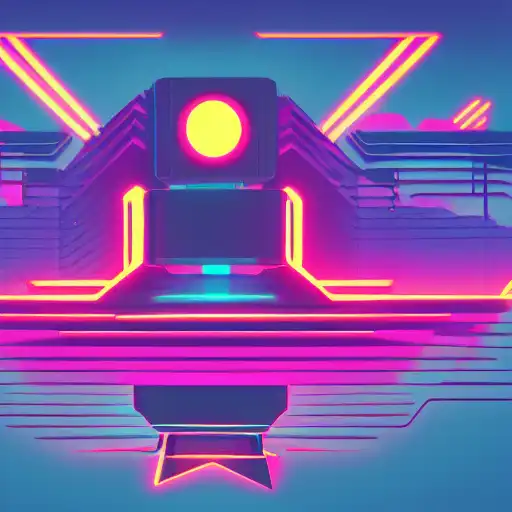
May 1st, 2023
Animations are a great way to add visual effects and improve the user experience in web applications. In Angular, animations are easy to create and use, thanks to the built-in support for animations. In this tutorial, we'll explore how to use animations in Angular.
Creating an Animation
To create an animation in Angular, we need to use the "AnimationBuilder" to create an animation definition. Let's create a simple animation for a button that fades in when the user hovers over it:
import { Component } from '@angular/core'; import { AnimationBuilder, style, animate } from '@angular/animations'; @Component({ selector: 'app-button', template: ` <button (mouseenter)="onHover()" (mouseleave)="onLeave()" [@fadeIn]="state">Hover Me!</button> `, animations: [ // Define the animation trigger trigger('fadeIn', [ state('void', style({ opacity: 0 })), state('*', style({ opacity: 1 })), transition('void => *', animate('500ms ease-in')), transition('* => void', animate('500ms ease-out')), ]), ], }) export class ButtonComponent { state = 'void'; constructor(private animationBuilder: AnimationBuilder) {} onHover() { const animation = this.animationBuilder.build([ animate('500ms ease-in', style({ opacity: 1 })), ]); animation.create(this.button.nativeElement).play(); this.state = '*'; } onLeave() { const animation = this.animationBuilder.build([ animate('500ms ease-out', style({ opacity: 0 })), ]); animation.create(this.button.nativeElement).play(); this.state = 'void'; } }
In this example, we're defining an animation trigger called "fadeIn". The trigger defines two states: "void" and "*", and two transitions: "void => " and " => void". The transitions specify the animation to use when transitioning between states.
We're also using the "AnimationBuilder" to create an animation definition for the "onHover" and "onLeave" methods. When the user hovers over the button, we're using the "create" method of the animation definition to play the animation on the button.
Using Animations
Now that we have our animation definition, let's use it in a component. We'll create a simple component that uses the "ButtonComponent" to display a button that fades in when the user hovers over it:
import { Component } from '@angular/core'; import { ButtonComponent } from './button.component'; @Component({ selector: 'app-root', template: ` <h1>My App</h1> <app-button></app-button> `, entryComponents: [ButtonComponent], }) export class AppComponent {}
In this example, we're using the "ButtonComponent" to display a button that fades in when the user hovers over it. We're also using the "entryComponents" property to define the "ButtonComponent" as a dynamic component.
Conclusion
In this tutorial, we've explored how to use animations in Angular. Animations are an essential part of most web applications, and Angular provides a powerful and flexible way to create and use animations using the "AnimationBuilder" and the "trigger" function. By defining animation triggers and using the "create" method of the animation definition, we can create and play animations in our components. In the next tutorial, we'll explore how to use Angular's built-in services to share data between components.