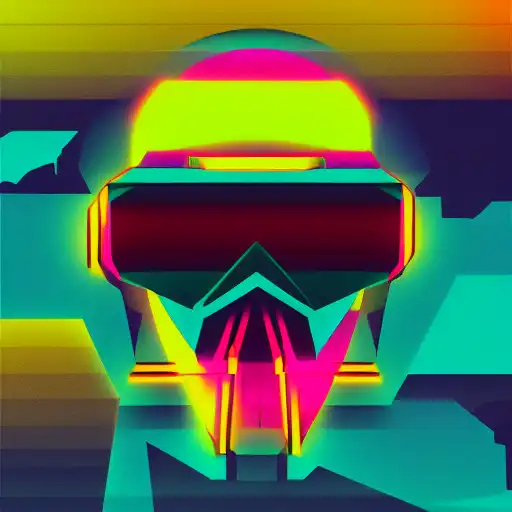
Apr 30th, 2023
Routing is an essential part of most web applications. In Angular, routing is easy to create and use, thanks to the built-in support for the "RouterModule". In this tutorial, we'll explore how to create and use routing in Angular.
Setting up Routing
To use routing in Angular, we need to import the "RouterModule" and define the routes for our application. Let's modify the "AppModule" to include routing:
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { RouterModule, Routes } from '@angular/router'; import { AppComponent } from './app.component'; import { HomeComponent } from './home.component'; import { AboutComponent } from './about.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, ]; @NgModule({ imports: [BrowserModule, RouterModule.forRoot(routes)], declarations: [AppComponent, HomeComponent, AboutComponent], bootstrap: [AppComponent], }) export class AppModule {}
In this example, we're defining two routes: the default route that displays the "HomeComponent", and a route for the "/about" URL that displays the "AboutComponent". We're also importing the "RouterModule" and using the "forRoot" method to configure our routes.
Creating Components
Now that we have our routes set up, let's create the components for our routes. We'll create a simple "HomeComponent" and "AboutComponent":
import { Component } from '@angular/core'; @Component({ selector: 'app-home', template: '<p>Welcome to the home page!</p>', }) export class HomeComponent {}
import { Component } from '@angular/core'; @Component({ selector: 'app-about', template: '<p>Welcome to the about page!</p>', }) export class AboutComponent {}
In these examples, we're defining two components: "HomeComponent" and "AboutComponent". These components are simple and only contain a basic template.
Using Router Links
Now that we have our routes and components set up, let's modify the "AppComponent" to use router links:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>My App</h1> <nav> <a routerLink="/">Home</a> <a routerLink="/about">About</a> </nav> <router-outlet></router-outlet> `, }) export class AppComponent {}
In this example, we're using the "routerLink" directive to create links to the "HomeComponent" and "AboutComponent". We're also using the "router-outlet" directive to display the active component based on the current route.
Conclusion
In this tutorial, we've explored how to create and use routing in Angular. Routing is an essential part of most web applications, and Angular provides a powerful and flexible way to create and use routes using the "RouterModule". By using router links and the router outlet directive, we can create a navigation menu and display the active component based on the current route. In the next tutorial, we'll explore how to use Angular's built-in animations to add visual effects to our application.