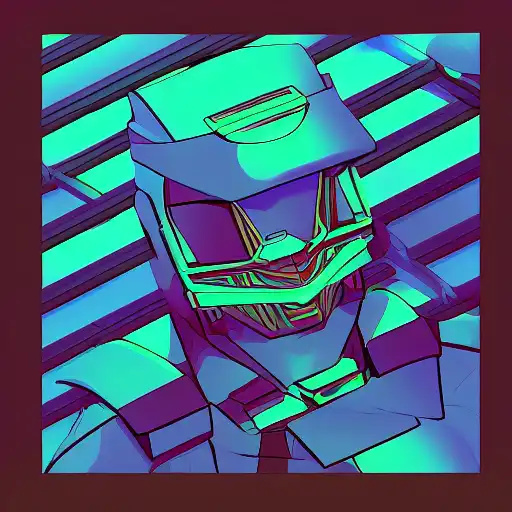
Apr 29th, 2023
Forms are an essential part of most web applications. In Angular, forms are easy to create and use, thanks to the built-in support for reactive forms. In this tutorial, we'll explore how to create and use forms in Angular.
Creating a Form
To create a form in Angular, we need to use the "FormsModule" or "ReactiveFormsModule". The "FormsModule" is used for template-driven forms, while the "ReactiveFormsModule" is used for reactive forms. In this example, we'll use the "ReactiveFormsModule" to create a simple form.
First, we need to import the "ReactiveFormsModule" module in the "AppModule":
import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ imports: [BrowserModule, ReactiveFormsModule], declarations: [AppComponent], bootstrap: [AppComponent], }) export class AppModule {}
Next, we can create a new component called "FormComponent" and add a form to its template:
import { Component } from '@angular/core'; import { FormBuilder } from '@angular/forms'; @Component({ selector: 'app-form', template: ` <form [formGroup]="form" (ngSubmit)="onSubmit()"> <label> Name: <input type="text" formControlName="name" /> </label> <br /> <label> Email: <input type="email" formControlName="email" /> </label> <br /> <button type="submit">Submit</button> </form> `, }) export class FormComponent { form = this.formBuilder.group({ name: '', email: '', }); constructor(private formBuilder: FormBuilder) {} onSubmit() { console.log(this.form.value); } }
In this example, we're using the "FormBuilder" to create a new form group with two form controls: "name" and "email". We're also binding the form group to the "form" property of the component and using the "ngSubmit" directive to handle the form submission.
Using Form Validation
Forms are often used to collect data from users, and it's important to validate that data before submitting it to the server. In Angular, form validation is easy to implement using validators.
Let's modify the "FormComponent" from the previous example to add some basic validation:
import { Component } from '@angular/core'; import { FormBuilder, Validators } from '@angular/forms'; @Component({ selector: 'app-form', template: ` <form [formGroup]="form" (ngSubmit)="onSubmit()"> <label> Name: <input type="text" formControlName="name" /> </label> <br /> <div *ngIf="name.invalid && (name.dirty || name.touched)"> <div *ngIf="name.errors.required">Name is required.</div> </div> <label> Email: <input type="email" formControlName="email" /> </label> <br /> <div *ngIf="email.invalid && (email.dirty || email.touched)"> <div *ngIf="email.errors.required">Email is required.</div> <div *ngIf="email.errors.email">Email must be a valid email address.</div> </div> <button type="submit">Submit</button> </form> `, }) export class FormComponent { form = this.formBuilder.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]], });
In this example, we're using the "Validators" module to add some basic validation to the "name" and "email" form controls. We're also using the "ngIf" directive to display error messages if the form controls are invalid and have been touched or modified.
Conclusion
In this tutorial, we've explored how to create and use forms in Angular. Forms are an essential part of most web applications, and Angular provides a powerful and flexible way to create and validate forms using the "ReactiveFormsModule". In the next tutorial, we'll explore how to use routing in Angular to navigate between different views of our application.