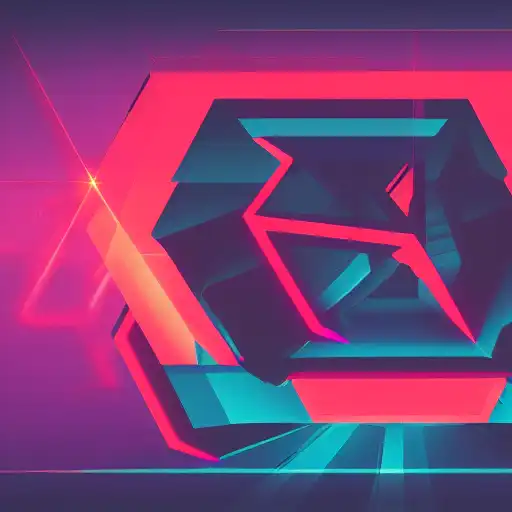
Apr 28th, 2023
In modern web applications, it's common to retrieve data from a server using HTTP. In Angular, the HttpClient module provides a powerful way to send HTTP requests and handle responses. In this tutorial, we'll explore how to use HttpClient to retrieve data from a server in Angular.
Creating a Simple API
Before we can retrieve data from a server, we need to create a simple API to serve the data. Let's create a simple API using Node.js and Express. First, create a new directory for the API and navigate into it:
mkdir my-api cd my-api
Next, initialize a new Node.js project and install Express:
npm init -y npm install express
Create a new file called "server.js" and add the following code:
const express = require('express'); const app = express(); const data = [ { id: 1, name: 'Item 1', price: 10 }, { id: 2, name: 'Item 2', price: 20 }, { id: 3, name: 'Item 3', price: 30 }, ]; app.get('/api/data', (req, res) => { res.json(data); }); app.listen(3000, () => { console.log('API server started on port 3000'); });
This code defines a simple API with a single route that returns an array of data. The API will be started on port 3000.
To start the API, run the following command:
node server.js
Retrieving Data from the API
Now that we have an API that serves data, let's modify the "MyService" from the previous example to use HttpClient to retrieve the data. First, we need to import the HttpClient module in "MyService":
import { HttpClient } from '@angular/common/http';
Next, we need to inject the HttpClient module into the "MyService" constructor:
constructor(private http: HttpClient) {}
Finally, we can use the "http.get" method to retrieve the data from the API:
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Injectable({ providedIn: 'root', }) export class MyService { private apiUrl = 'http://localhost:3000/api/data'; constructor(private http: HttpClient) {} getData() { return this.http.get(this.apiUrl); } }
In this example, we're defining a new method called "getData" that uses the "http.get" method to retrieve the data from the API. We're also defining a private "apiUrl" property that stores the URL of the API.
Now, let's modify the "ChildComponent" from the previous example to use the "MyService" to retrieve the data:
import { Component } from '@angular/core'; import { MyService } from './my-service.service'; @Component({ selector: 'app-child', template: ` <p>Child Component</p> <ul> <li *ngFor="let item of items">{{ item.name }} - ${{ item.price }}</li> </ul> `, }) export class ChildComponent { items: any[] = []; constructor(private myService: MyService) {} ngOnInit() { this.myService.getData().subscribe((data: any) => { this.items = data; }); } }
In this example, we're injecting the "MyService" service into the "ChildComponent" constructor. We are also using the "ngOnInit" lifecycle hook to retrieve the data from the API using the "getData" method of the service. We're then using the "subscribe" method to handle the asynchronous response from the API and store the data in the "items" property.
Conclusion
In this tutorial, we've explored how to use HttpClient to retrieve data from a server in Angular. By creating a simple API and modifying the "MyService" to use HttpClient, we were able to retrieve data and display it in a component. Using HTTP is an essential feature of modern web applications, and Angular provides a powerful and flexible way to work with HTTP requests and responses. In the next tutorial, we'll explore how to use forms in Angular to allow users to input data into our application.