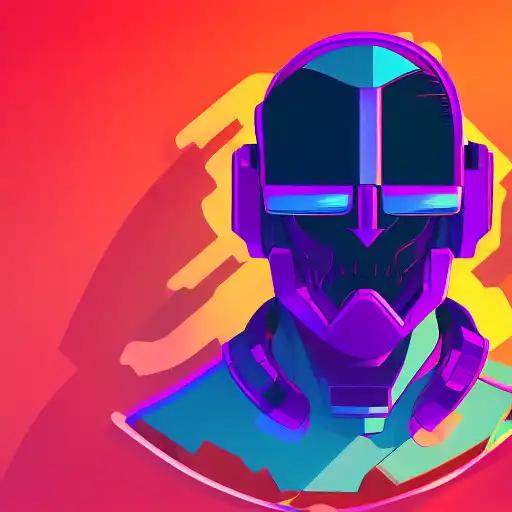
Apr 27th, 2023
In Angular, services are used to share data and functionality between components. Services provide a way to centralize functionality and make it available to multiple components. In this tutorial, we'll explore how to use services to share data between components.
Creating a Service
To create a service, you can use the Angular CLI command:
ng generate service my-service
This will create a new service called "my-service" in your project's "src/app" directory. It will contain a TypeScript file for the service's logic.
Let's create a simple service that provides a method for getting and setting a message:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class MyService { private message: string = ''; setMessage(message: string) { this.message = message; } getMessage(): string { return this.message; } }
In this example, we're creating a service called "MyService" that has two methods: "setMessage" and "getMessage". The "setMessage" method sets the "message" property to the provided value, and the "getMessage" method returns the current value of the "message" property.
Using a Service
To use a service in a component, you need to inject it into the component's constructor. Let's modify the "ParentComponent" from the previous example to use the "MyService" service:
import { Component } from '@angular/core'; import { MyService } from './my-service.service'; @Component({ selector: 'app-parent', template: ` <p>Parent Component</p> <button (click)="setMessage()">Set Message</button> <app-child></app-child> `, }) export class ParentComponent { constructor(private myService: MyService) {} setMessage() { this.myService.setMessage('Hello from parent!'); } }
In this example, we're injecting the "MyService" service into the "ParentComponent" constructor. We're also adding a button that calls the "setMessage" method of the service when clicked.
Now, let's modify the "ChildComponent" to use the "MyService" service to get the message:
import { Component } from '@angular/core'; import { MyService } from './my-service.service'; @Component({ selector: 'app-child', template: ` <p>Child Component</p> <p>{{ message }}</p> `, }) export class ChildComponent { message: string = ''; constructor(private myService: MyService) {} ngOnInit() { this.message = this.myService.getMessage(); } }
In this example, we're injecting the "MyService" service into the "ChildComponent" constructor. We're also using the "ngOnInit" lifecycle hook to get the message from the service and store it in the "message" property.
Conclusion
Using services to share data and functionality between components in Angular is a powerful feature that allows you to create more complex and interactive applications. By centralizing functionality in a service, you can make it available to multiple components and avoid code duplication. In the next tutorial, we'll explore how to use HTTP to retrieve data from a server in Angular.