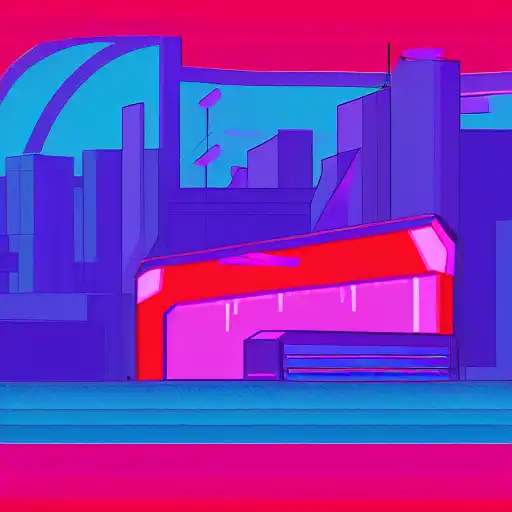
Apr 26th, 2023
In Angular, components are the building blocks of web applications. They allow you to encapsulate your code into smaller, reusable parts that can be combined to create more complex applications. One of the key features of components is the ability to communicate with each other by passing data between them. In this tutorial, we'll explore how to pass data between components using inputs and outputs.
Inputs and Outputs
Inputs and outputs are two mechanisms for passing data between components in Angular.
An input is a way to pass data from a parent component to a child component. To create an input in a component, you need to use the @Input decorator. Let's create a new component called "parent" and a child component called "child". Here's an example of how to pass data from the parent component to the child component using inputs:
import { Component, Input } from '@angular/core'; @Component({ selector: 'app-child', template: ` <p>Child Component</p> <p>{{ data }}</p> `, }) export class ChildComponent { @Input() data: string; } @Component({ selector: 'app-parent', template: ` <p>Parent Component</p> <app-child [data]="message"></app-child> `, }) export class ParentComponent { message = 'Hello, child!'; }
In this example, we're creating a child component that receives data through an input property called "data". The parent component creates an instance of the child component and sets the "data" property to the value of the "message" property. The child component then displays the value of the "data" property in its template.
Outputs, on the other hand, allow a child component to emit events that a parent component can listen to. To create an output in a component, you need to use the @Output decorator. Here's an example of how to use outputs to send a message from a child component to a parent component:
import { Component, Output, EventEmitter } from '@angular/core'; @Component({ selector: 'app-child', template: ` <button (click)="sendMessage()">Send Message</button> `, }) export class ChildComponent { @Output() messageEvent = new EventEmitter<string>(); sendMessage() { this.messageEvent.emit('Hello from child!'); } } @Component({ selector: 'app-parent', template: ` <p>Parent Component</p> <app-child (messageEvent)="receiveMessage($event)"></app-child> <p>{{ message }}</p> `, }) export class ParentComponent { message = ''; receiveMessage(message: string) { this.message = message; } }
In this example, we're creating a child component with a button that emits a message through an output property called "messageEvent". The parent component creates an instance of the child component and listens to the "messageEvent" event using the (messageEvent) binding. When the child component emits the "messageEvent" event, the parent component receives the message and updates the "message" property, which is displayed in the template.
Conclusion
Passing data between components in Angular is a powerful feature that allows you to build more complex and interactive applications. By using inputs and outputs, you can create a more modular and reusable codebase. In the next tutorial, we'll explore how to use services to share data between components.