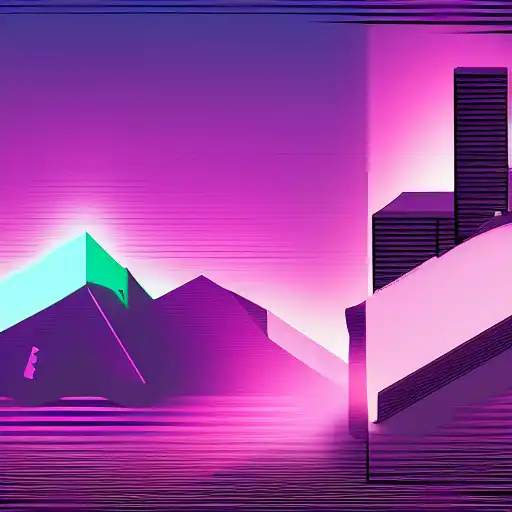
Apr 25th, 2023
Components are the building blocks of Angular applications. They are responsible for rendering the user interface and responding to user events. In this tutorial, we'll explore how to create and use components in Angular.
To create a new component, use the Angular CLI command:
ng generate component my-component
This will create a new directory called "my-component" in your project's "src/app" directory. It will contain several files, including a TypeScript file for the component's logic, an HTML file for its template, and a CSS file for its styles.
Now, let's modify the component's template to display some content. Open the "my-component.component.html" file and add the following code:
<h1>Hello, world!</h1>
Save the file and return to the terminal. Run the application using the following command:
ng serve --open
Your browser will automatically open, and you should see the "Hello, world!" text displayed on the screen.
Next, let's add some logic to the component. Open the "my-component.component.ts" file and add the following code to the class definition:
export class MyComponentComponent implements OnInit { constructor() { } ngOnInit(): void { console.log('Component initialized'); } }
This code defines a new method called "ngOnInit", which is a lifecycle hook that runs when the component is initialized. In this case, it logs a message to the console.
Finally, let's add the component to the application's main template. Open the "app.component.html" file and replace its contents with the following code:
<app-my-component></app-my-component>
This code inserts the "my-component" into the main application template. Save the file and return to the terminal to run the application again. You should now see the "Hello, world!" text and a message in the console indicating that the component was initialized.
Congratulations, you've successfully created and used a component in Angular! In the next tutorial, we'll explore how to pass data between components using inputs and outputs.
In conclusion, components are essential to building Angular applications. They define the user interface and contain the application logic. By following these steps, you can create and use components in your Angular applications.