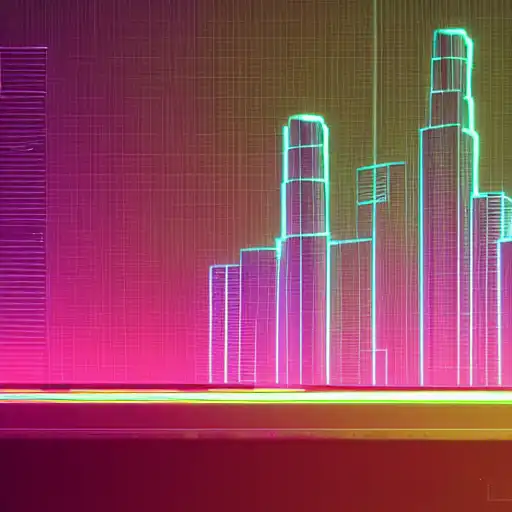
Apr 20th, 2023
In the previous post, we showed you how to create a web server using Spring Boot and implement a simple REST API for managing notes. In this post, we'll explore how to use Spring Security to secure your Java web application.
Spring Security is a powerful and highly customizable security framework for Java web applications. It provides a wide range of security features, including authentication, authorization, and encryption.
Step 1: Add Spring Security to Your Project
To add Spring Security to your project, you can add the following dependency to your pom.xml file:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
Step 2: Configure Spring Security
To configure Spring Security, you can create a new class that extends the WebSecurityConfigurerAdapter class and overrides its configure method. In this method, you can define the security rules for your application.
For example, here's a simple configuration that requires authentication for all requests:
package com.example.notes; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; @Configuration @EnableWebSecurity public class SecurityConfiguration extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .anyRequest().authenticated() .and() .formLogin() .and() .logout(); } }
This configuration requires authentication for all requests and provides a default login form and logout functionality.
Step 3: Test Your Security Configuration
To test your security configuration, you can try accessing your REST API endpoints without logging in. You should see a "401 Unauthorized" response. After logging in, you should be able to access the endpoints successfully.
Congratulations! You've successfully secured your Java web application using Spring Security. In the next post, we'll explore how to use Hibernate to store and retrieve data from a MySQL database.