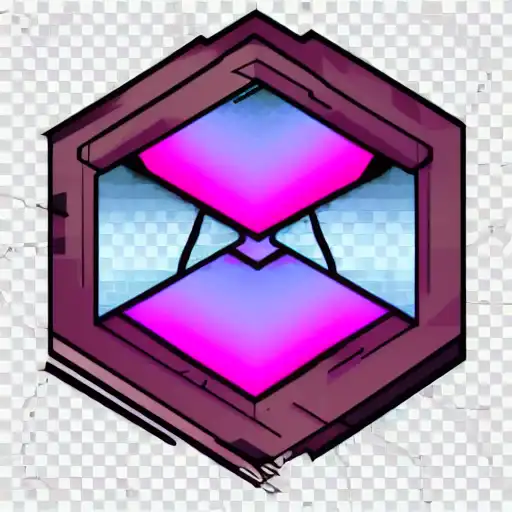
Apr 21th, 2023
In the previous post, we showed you how to use Spring Security to secure your Java web application. In this post, we'll explore how to use Hibernate to store and retrieve data from a MySQL database.
Hibernate is a popular Object-Relational Mapping (ORM) tool for Java that provides a simple and efficient way to map Java objects to relational database tables. It also provides powerful query capabilities and caching mechanisms that can improve the performance of your database operations.
Step 1: Create a MySQL Database
To create a MySQL database for this example, you can use the MySQL Workbench application or the MySQL command line interface. Create a new database named "notes" with a user named "notesuser" and a password of your choice.
Step 2: Add Hibernate and MySQL Dependencies
To add Hibernate and MySQL dependencies to your project, you can add the following dependencies to your pom.xml file:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
Step 3: Configure Hibernate
To configure Hibernate, you can create a new properties file named "application.properties" in the "src/main/resources" directory and add the following lines:
spring.datasource.url=jdbc:mysql://localhost:3306/notes spring.datasource.username=notesuser spring.datasource.password=password spring.jpa.hibernate.ddl-auto=create
This configuration sets the database URL, username, and password, and specifies that Hibernate should create the necessary database tables automatically.
Step 4: Create a Note Entity
To create a Note entity, you can create a new Java class named "Note" in the "com.example.notes" package and add the following lines:
package com.example.notes; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Note { @Id @GeneratedValue(strategy = GenerationType.AUTO) private Long id; private String title; private String content; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getContent() { return content; } public void setContent(String content) { this.content = content; } }
This entity defines the database table structure for the "Note" object.
Step 5: Create a Note Repository
To create a Note repository, you can create a new Java interface named "NoteRepository" in the "com.example.notes" package and add the following lines:
package com.example.notes; import org.springframework.data.repository.CrudRepository; public interface NoteRepository extends CrudRepository<Note, Long> { }
This repository provides a set of methods for creating, reading, updating, and deleting Note objects in the database.
Step 6: Test Your Hibernate Configuration
To test your Hibernate configuration, you can modify the NoteController class from the previous post to use the NoteRepository for storing and retrieving Note objects from the database:
package com.example.notes; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.httprequestMapping("/notes") public class NoteController { @Autowired private NoteRepository noteRepository; @GetMapping("/{id}") public ResponseEntity<Note> getNoteById(@PathVariable("id") Long id) { Note note = noteRepository.findById(id).orElse(null); if (note == null) { return ResponseEntity.notFound().build(); } else { return ResponseEntity.ok(note); } } @PostMapping("") public ResponseEntity<Note> createNote(@RequestBody Note note) { Note savedNote = noteRepository.save(note); return ResponseEntity.status(HttpStatus.CREATED).body(savedNote); } @PutMapping("/{id}") public ResponseEntity<Note> updateNote(@PathVariable("id") Long id, @RequestBody Note note) { Note existingNote = noteRepository.findById(id).orElse(null); if (existingNote == null) { return ResponseEntity.notFound().build(); } else { existingNote.setTitle(note.getTitle()); existingNote.setContent(note.getContent()); Note updatedNote = noteRepository.save(existingNote); return ResponseEntity.ok(updatedNote); } } @DeleteMapping("/{id}") public ResponseEntity<Void> deleteNoteById(@PathVariable("id") Long id) { noteRepository.deleteById(id); return ResponseEntity.noContent().build(); }
You can then run the application using the "mvn spring-boot:run" command and test the REST API endpoints to ensure that Note objects are being stored and retrieved from the database correctly.
Congratulations! You've successfully used Hibernate to store and retrieve data from a MySQL database. In the next post, we'll explore how to use Thymeleaf to create dynamic HTML templates for your Java web application.