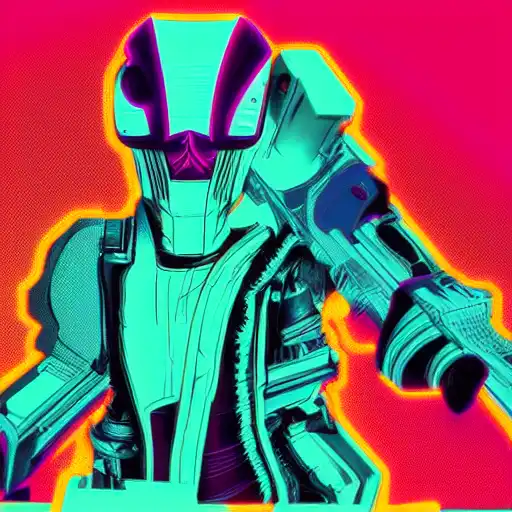
Apr 19th, 2023
In the previous post, we showed you how to use Spring Boot to simplify the deployment process for your Java application. In this post, we'll provide an example program for a web server using Spring Boot.
This example program is a simple REST API that allows users to create, read, update, and delete notes. It uses Spring Boot, Spring Data JPA, and H2 as an in-memory database.
Step 1: Create a Spring Boot Project
To create a Spring Boot project for this example, you can use the Spring Initializr website or the Spring Tool Suite plugin for IntelliJ. Choose "Web" as the project type and select the following dependencies:
- Spring Web
- Spring Data JPA
- H2 Database
Step 2: Write Your Java Code
In the editor window, create a new package named "com.example.notes" and create the following classes:
package com.example.notes; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; @Entity public class Note { @Id @GeneratedValue(strategy = GenerationType.AUTO) private Long id; private String title; private String content; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getContent() { return content; } public void setContent(String content) { this.content = content; } }
package com.example.notes; import org.springframework.data.repository.CrudRepository; public interface NoteRepository extends CrudRepository{ }
package com.example.notes; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.http.HttpStatus; import org.springframework.http.ResponseEntity; import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/notes") public class NoteController { @Autowired private NoteRepository noteRepository; @GetMapping("/{id}") public ResponseEntitygetNoteById(@PathVariable("id") Long id) { Note note = noteRepository.findById(id).orElse(null); if (note == null) { return ResponseEntity.notFound().build(); } else { return ResponseEntity.ok(note); } } @PostMapping("") public ResponseEntity createNote(@RequestBody Note note) { Note savedNote = noteRepository.save(note); return ResponseEntity.status(HttpStatus.CREATED).body(savedNote); } @PutMapping("/{id}") public ResponseEntity updateNote(@PathVariable("id") Long id, @RequestBody Note note) { Note existingNote = noteRepository.findById(id).orElse(null); if (existingNote == null) { return ResponseEntity.notFound().build(); } else { existingNote.setTitle(note.getTitle()); existingNote.setContent(note.getContent()); Note updatedNote = noteRepository.save(existingNote); return ResponseEntity.ok(updatedNote); } } @DeleteMapping("/{id}") public ResponseEntity deleteNoteById(@PathVariable("id") Long id) { noteRepository.deleteById(id); return ResponseEntity.noContent().build(); } }
Step 3: Build and Run Your Application
To build and run your Spring Boot application, open a terminal window and navigate to your project directory. Then run the following command:
mvn spring-boot:run
This will compile your Java code, download any necessary dependencies, and start your Spring Boot application. You can access the REST API endpoints by navigating to http://localhost:8080/notes in your web browser.
Step 4: Test Your REST API
To test your REST API, you can use a tool like Postman or curl to make HTTP requests to the API endpoints. Here are some examples of HTTP requests you can make:
- GET /notes/{id} - Retrieves the note with the specified ID.
- POST /notes - Creates a new note with the specified title and content.
- PUT /notes/{id} - Updates the note with the specified ID with the new title and content.
- DELETE /notes/{id} - Deletes the note with the specified ID.
Congratulations! You've successfully created a web server using Spring Boot and implemented a simple REST API for managing notes. In the next post, we'll explore how to use Spring Security to secure your Java web application.