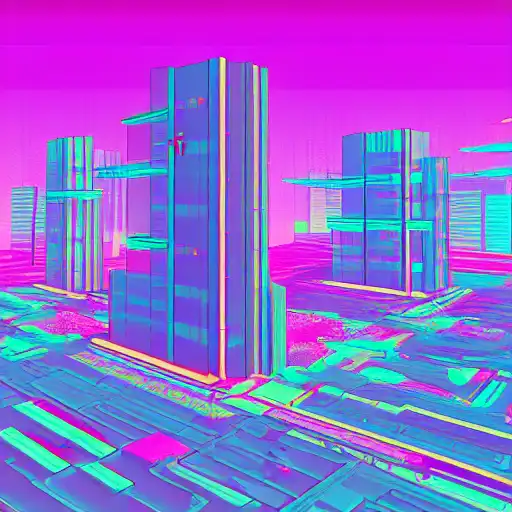
Apr 18th, 2023
In the previous post, we showed you how to deploy your Java application to a web server using Maven and Tomcat. In this post, we'll explore how to use Spring Boot to simplify the deployment process for your Java application.
Spring Boot is a popular framework for building Java applications that can be easily deployed to a web server. It provides a set of libraries and conventions that simplify the development process and reduce the amount of boilerplate code required.
Step 1: Create a Spring Boot Project
To create a Spring Boot project, you can use the Spring Initializr website or the Spring Tool Suite plugin for IntelliJ. Choose "Web" as the project type and select any necessary dependencies for your project, such as Spring Web, Spring Data JPA, and MySQL.
Step 2: Write Your Java Code
In the editor window, write your Java code as you would for any Java project. However, with Spring Boot, you can take advantage of features such as automatic configuration and dependency injection to simplify your code.
For example, here's a simple Spring Boot application that responds to HTTP requests with a message:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class HelloWorldApplication { public static void main(String[] args) { SpringApplication.run(HelloWorldApplication.class, args); } @GetMapping("/") public String helloWorld() { return "Hello, world!"; } }
This code defines a Spring Boot application with a single endpoint ("/") that responds to HTTP GET requests with the message "Hello, world!"
Step 3: Build and Run Your Application
To build and run your Spring Boot application, open a terminal window and navigate to your project directory. Then, run the following command:
mvn spring-boot:run
This will compile your Java code, download any necessary dependencies, and start your Spring Boot application. You can access your application by navigating to http://localhost:8080 in your web browser.
Step 4: Package and Deploy Your Application
To package your Spring Boot application into a deployable JAR file, run the following command:
mvn package
This will create a JAR file in the "target" directory that contains your application and all necessary dependencies.
To deploy your Spring Boot application to a web server, you can simply copy the JAR file to the server and run it using the "java -jar" command. Spring Boot provides built-in support for many popular web servers, such as Tomcat and Jetty, and can also be deployed to cloud platforms like Heroku and AWS.
Congratulations! You've successfully used Spring Boot to simplify the deployment process for your Java application. In the next post, we'll provide an example program for a web server using Spring Boot.