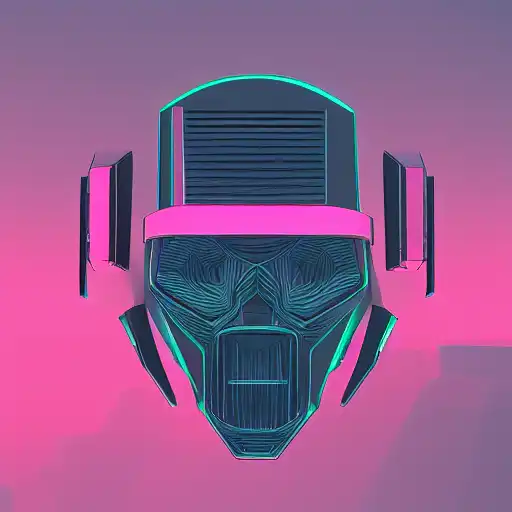
Apr 17th, 2023
In the previous post, we showed you how to use continuous integration with Jenkins to automate your build and test process for your Java project. In this post, we'll guide you through the process of deploying your Java application to a web server using Maven and Tomcat on Linux.
Step 1: Install Tomcat
To install Tomcat on your Linux system, you can follow the official Tomcat installation guide for Linux. Once Tomcat is installed, you can access the Tomcat web interface by navigating to http://localhost:8080 in your web browser.
Step 2: Configure Your Maven Project
To deploy your Java application to Tomcat using Maven, you need to add the Tomcat Maven plugin to your project. Open the pom.xml file in IntelliJ and add the following lines to the build/plugins section:
<plugins> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <version>2.2</version> <configuration> <url>http://localhost:8080/manager/text</url> <server>TomcatServer</server> <path>/myapp</path> </configuration> </plugin> </plugins>
This will add the Tomcat Maven plugin to your project and configure it to deploy your application to Tomcat.
Step 3: Configure Your Tomcat Server
To deploy your Java application to Tomcat, you need to configure a user with the "manager-script" role in Tomcat. Open the tomcat-users.xml file in your Tomcat installation directory and add the following lines:
<user username="admin" password="password" roles="manager-gui,manager-script,admin-gui"/>
This will create a new user with the username "admin" and password "password" with the necessary roles to deploy your application.
Step 4: Deploy Your Application
To deploy your Java application to Tomcat using Maven, open a terminal window and navigate to your project directory. Then, run the following command:
mvn tomcat7:deploy
This will deploy your application to Tomcat and start it. You can access your application by navigating to http://localhost:8080/myapp in your web browser.
Step 5: Undeploy Your Application
To undeploy your Java application from Tomcat using Maven, run the following command:
mvn tomcat7:undeploy
This will undeploy your application from Tomcat.
Congratulations! You've successfully deployed your Java application to a web server using Maven and Tomcat on Linux. In the next post, we'll explore how to use Spring Boot to simplify the deployment process for your Java application.