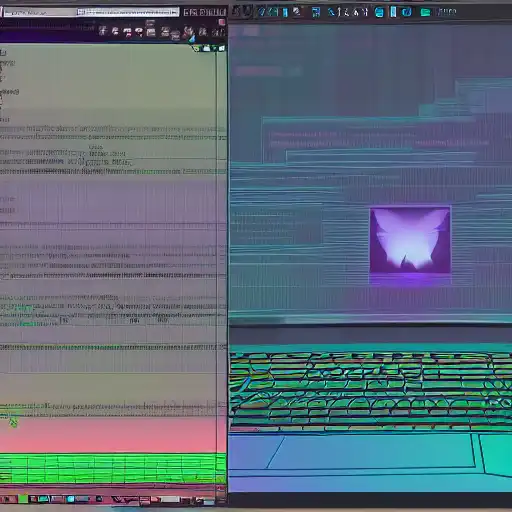
Mar 14th, 2023
Racket's Support for Error Handling
In this blog post, we will explore Racket's support for error handling and exception handling, including how to handle errors and exceptions gracefully in your Racket programs.
Racket provides a variety of built-in mechanisms for handling errors and exceptions. These mechanisms allow you to catch and handle errors that might occur during the execution of your program, and to gracefully recover from those errors or exit the program cleanly.
Racket's Error Mechanisms
Racket provides several built-in error mechanisms that you can use to report errors and exceptions in your programs.
The most common error mechanism is error
, which takes a string message and raises an error with that message.
Here's an example of using error
in Racket:
#lang racket (define (my-function arg) (if (not (string? arg)) (error "my-function expects a string argument") ; rest of the function implementation ))
In this example, we define a function called my-function
that expects a string argument.
If the argument is not a string, we raise an error with the message "my-function expects a string argument".
This error will cause the program to terminate, unless it is caught and handled by an exception handler.
Racket's Exception Handling
Racket provides a built-in with-handlers
form for catching and handling exceptions in your programs.
with-handlers
takes one or more exception handlers and a body of code to execute.
If an exception is raised during the execution of the code, the appropriate handler will be called to handle the exception.
Here's an example of using with-handlers
in Racket:
#lang racket (define (my-function arg) (with-handlers ([exn:fail? (lambda (exn) (error "my-function: an error occurred"))]) ; rest of the function implementation ))
In this example, we define a function called my-function
that wraps its implementation code in a with-handlers
form.
The exn:fail?
exception handler is used to catch any exceptions that might occur during the execution of the code.
If an exception is caught, the handler raises another error with the message "my-function: an error occurred".
Conclusion
Racket's built-in support for error handling and exception handling makes it easy to write programs that can gracefully recover from errors and exceptions.
The error function provides a simple way to raise errors when something goes wrong, while the with-handlers
form allows you to catch and handle exceptions in a controlled manner.
With Racket's support for error handling, you can write programs that are robust, reliable, and easy to maintain.