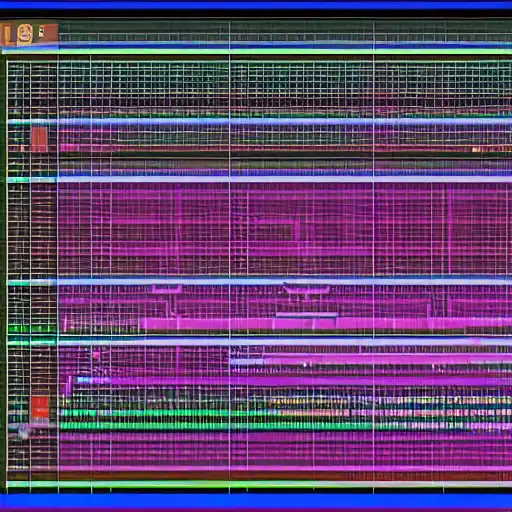
Mar 15th, 2023
Regular Expressions in Racket
In this blog post, we will explore Racket's support for regular expressions, including how to use them to search and manipulate text in your Racket programs.
A regular expression is a pattern that describes a set of strings. Regular expressions are widely used in text processing applications to search for and manipulate text. Racket provides a built-in library for working with regular expressions, which makes it easy to use regular expressions in your Racket programs.
Racket's regex
Library
Racket's regex
library provides a set of functions for working with regular expressions in Racket.
These functions allow you to compile regular expressions, match regular expressions against strings, and extract matching substrings from strings.
Here's an example of using regex
in Racket:
#lang racket (require racket/regexp) (define regex (regexp #rx"^hello, (.*)$")) (define (my-function str) (if (regexp-match? regex str) (let ((match (regexp-match regex str))) (match:get match 1)) "no match"))
In this example, we define a regular expression pattern that matches strings that start with the text "hello, " and have any text after it.
We then define a function called my-function
that takes a string argument and checks whether it matches the regular expression.
If it does, the function extracts the matching substring and returns it.
If it doesn't, the function returns the string "no match".
Regular Expression Syntax
Regular expressions have a syntax that allows you to specify patterns for matching strings. Here are some of the most commonly used elements of regular expression syntax:
.
matches any single character*
matches zero or more occurrences of the preceding character or group+
matches one or more occurrences of the preceding character or group?
matches zero or one occurrence of the preceding character or group|
separates alternative patterns()
groups characters or patterns together[]
matches any single character in the set of characters within the brackets[^]
matches any single character not in the set of characters within the brackets\
escapes the next character to allow it to be used as a literal character in the pattern
Conclusion
Racket's built-in support for regular expressions makes it easy to work with text in your Racket programs.
The regex
library provides a set of functions for compiling and using regular expressions in Racket.
With regular expressions, you can search for and manipulate text in your programs with ease, making your code more powerful and flexible.