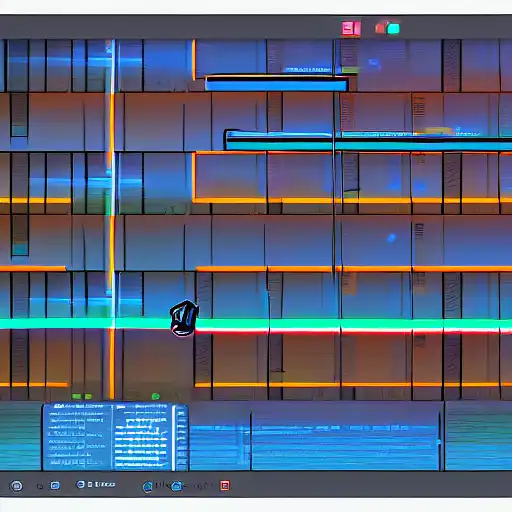
Mar 13th, 2023
Racket's Support for Testing
Racket provides a comprehensive framework for testing your code, including support for both unit testing and integration testing.
The framework is built on top of the rackunit
library, which provides a wide range of tools for testing and debugging your Racket programs.
Unit Testing with Racket
Racket's unit testing framework is based on the concept of a test case, which is a function that checks whether some aspect of your code behaves as expected. A test case typically consists of one or more assertions, which verify that a certain condition holds true. For example, you might write a test case that checks whether a function returns the correct output for a given input.
Here's an example of a simple test case in Racket:
#lang racket (require rackunit) (define (test-my-function) (check-equal? (my-function 2) 4) (check-equal? (my-function -3) 9))
In this example, we define a test case called test-my-function
that checks whether the my-function
function returns the correct output for two different inputs.
We use the check-equal?
assertion to compare the output of my-function
to the expected value.
Integration Testing with Racket
Integration testing is the process of testing how different components of your application work together.
In Racket, you can use the web-server/testing
library to write integration tests for your web applications.
Here's an example of a simple integration test in Racket:
#lang racket (require web-server/testing) (define (test-my-web-app) (with-handlers ([exn:fail? (lambda (exn) (void))]) (run-server-tests (list (test-post "/submit" "name=John&email=john@example.com" (λ (response) (check-equal? (response-status response) 200) (check-true (string-contains? (response-body/response response) "Thank you for submitting your information!")))) (test-get "/contact" (λ (response) (check-equal? (response-status response) 200) (check-true (string-contains? (response-body/response response) "Contact Us")))))))) (test-my-web-app)
In this example, we define an integration test called test-my-web-app
that tests two different endpoints of a web application.
We use the test-post
and test-get
functions to simulate HTTP requests to the /submit
and /contact
endpoints, respectively.
We use the check-equal?
and check-true
assertions to verify that the HTTP response contains the expected content.
Conclusion
Racket's built-in support for testing makes it easy to write robust and reliable code.
The rackunit
library provides a comprehensive set of tools for unit testing, while the web-server/testing
library provides a simple way to write integration tests for your web applications.
With Racket's support for testing, you can be confident that your code works as expected, even as it grows in complexity.