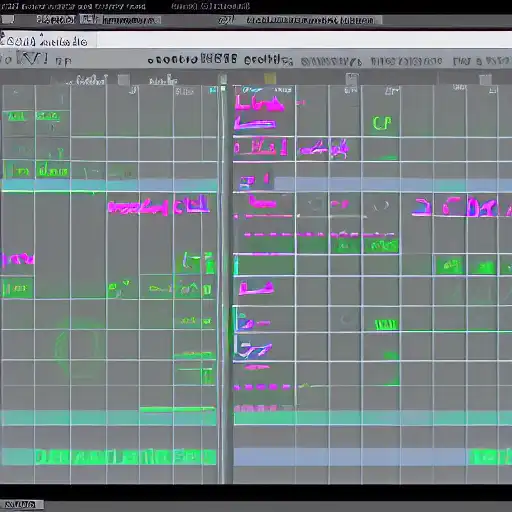
Mar 10th, 2023
Welcome back to our Racket series. In the previous post, we discussed Racket's support for object-oriented programming. In this post, we will explore Racket's support for concurrency and parallelism.
Concurrency and Parallelism
Concurrency and parallelism are two concepts that are often used interchangeably, but they have different meanings. Concurrency is the ability of a program to execute multiple tasks or threads simultaneously, while parallelism is the ability of a program to execute multiple tasks or threads simultaneously on different processors or cores.
Racket supports both concurrency and parallelism through its thread
and future
libraries.
Threads
A thread is a lightweight process that shares the same memory space as the main thread. Threads are useful for performing multiple tasks simultaneously.
Here's an example of a simple program that uses threads:
#lang racket (define (print-loop id) (for ([i 10]) (printf "Thread ~a: ~a\n" id i) (sleep 1))) (define t1 (thread (lambda () (print-loop "A")))) (define t2 (thread (lambda () (print-loop "B")))) (join-thread t1) (join-thread t2)
In this example, we define a function called print-loop
that prints numbers from 0
to 9
with a delay of one second between each number.
We create two threads, t1
and t2
, each of which calls the print-loop
function with a different ID.
Finally, we join the two threads so that the program waits for them to complete before exiting.
Futures
A future is a value that is computed asynchronously. Futures are useful for performing expensive computations in the background and retrieving the result when it is needed.
Here's an example of a simple program that uses futures:
#lang racket (define (fib n) (if (< n 2) n (+ (future (fib (- n 1))) (future (fib (- n 2)))))) (fib 30)
In this example, we define a function called fib
that calculates the Fibonacci sequence recursively.
We use futures to calculate the result of each recursive call asynchronously.
Finally, we call the fib
function with an argument of 30
.
Benefits of Concurrency and Parallelism
Concurrency and parallelism provide several benefits for writing efficient and responsive programs:
- Improved performance: Concurrency and parallelism can improve the performance of programs by allowing multiple tasks to be performed simultaneously.
- Responsiveness: Concurrency and parallelism can make programs more responsive by allowing them to handle multiple user requests simultaneously.
- Resource utilization: Concurrency and parallelism can make more efficient use of system resources, such as CPUs and memory.
Conclusion
In this post, we explored Racket's support for concurrency and parallelism. We provided examples of using threads and futures to perform tasks simultaneously and asynchronously, respectively. We also discussed the benefits of concurrency and parallelism, including improved performance, responsiveness, and resource utilization. In the next post, we will discuss Racket's support for functional programming.