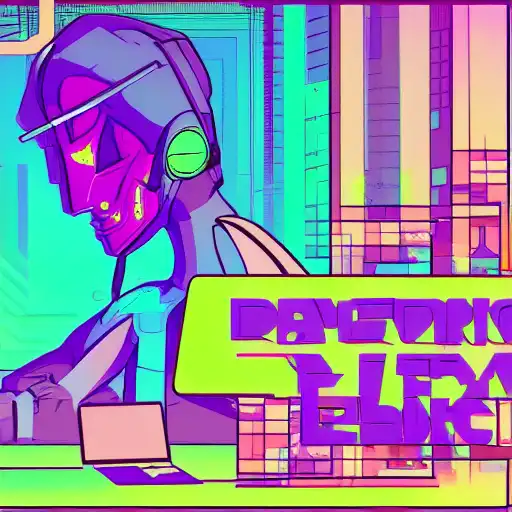
Mar 11th, 2023
In this post, we'll take a deeper dive into Racket's support for functional programming. We've already seen some examples of functional programming in previous posts, but here we'll discuss the core concepts and techniques of functional programming in more detail.
Functional Programming Basics
Functional programming is a programming paradigm that emphasizes the use of functions to create programs. Functional programming treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Racket provides built-in support for functional programming, making it a powerful tool for developing functional applications.
Functions
Functions are the building blocks of functional programming. In Racket, functions are first-class objects, meaning they can be passed as arguments to other functions, returned as values, and stored in variables.
Here's an example of a simple function in Racket:
#lang racket (define (square x) (* x x)) (square 5) ; Returns 25
In this example, we define a function called square
that takes a single argument x
and returns the square of x
.
We then call the square
function with an argument of 5
, which returns 25
.
Higher-order Functions
Higher-order functions are functions that take other functions as arguments or return functions as values. Higher-order functions are a powerful tool in functional programming because they allow us to abstract common patterns of computation.
Here's an example of a higher-order function in Racket:
#lang racket (define (apply-twice f x) (f (f x))) (apply-twice square 5) ; Returns 625
In this example, we define a function called apply-twice
that takes two arguments, a function f
and a value x
.
The apply-twice
function applies f
to x
twice and returns the result.
We call the apply-twice
function with the square
function and an argument of 5
, which returns 625
.
Lambda Expressions
Lambda expressions are anonymous functions that can be used to define functions on-the-fly. Lambda expressions are useful when we need to define a function that is used only once.
Here's an example of a lambda expression in Racket:
#lang racket ((lambda (x) (* x x)) 5) ; Returns 25
In this example, we define a lambda expression that takes a single argument x
and returns the square of x
.
We then call the lambda expression with an argument of 5
, which returns 25
.
Closures
Closures are functions that capture the values of their free variables. Closures are useful for defining functions that depend on values that are not passed as arguments.
Here's an example of a closure in Racket:
#lang racket (define (make-adder x) (lambda (y) (+ x y))) (define add5 (make-adder 5)) (add5 10) ; Returns 15
In this example, we define a function called make-adder
that takes a single argument x
and returns a closure that adds x
to its argument.
We then define a closure called add5
that adds 5
to its argument.
We call add5
with an argument of 10
, which returns 15
.
Immutable Data
In functional programming, data is often immutable, meaning it cannot be changed after it is created. Immutable data is useful for creating data structures that are efficient and easy to reason about.
Racket provides several built-in immutable data structures, including lists, vectors, and hash tables.
Here's an example of an immutable data structure in Racket:
#lang racket (define my-list '(1 2)) (define my-new-list (cons 3 my-list)) my-list ; Returns '(1 2) my-new-list ; Returns '(3 1 2)
In this example, we define an immutable list called my-list
that contains the elements 1
and 2
.
We then use the `cons` function to create a new list called my-new-list
that contains the element 3
followed by the elements of my-list
.
We print the values of my-list
and my-new-list
to the console, which show that my-list
is still unchanged and my-new-list
contains the additional element 3
.
Conclusion
Functional programming is a powerful programming paradigm that emphasizes the use of functions to create programs. Racket provides built-in support for functional programming, making it a great tool for developing functional applications. In this post, we discussed the basics of functional programming in Racket, including functions, higher-order functions, lambda expressions, closures, and immutable data. By understanding these concepts, you can write efficient and easy-to-reason-about code in Racket.