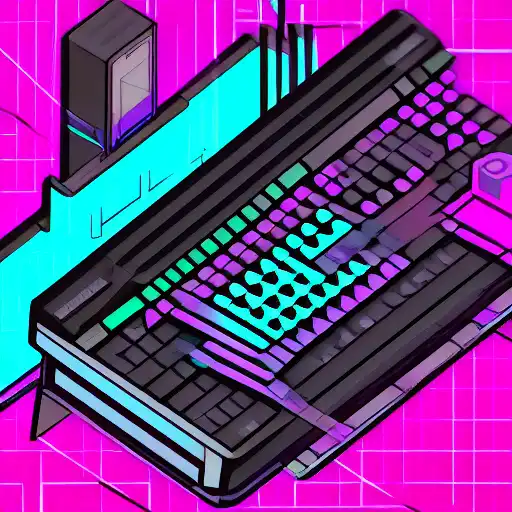
Mar 9th, 2023
Welcome back to our Racket series. In the previous post, we discussed Racket's module system and its benefits for organizing code. In this post, we will explore Racket's support for object-oriented programming.
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects, which are instances of classes that encapsulate data and behavior. OOP provides several benefits, including modularity, encapsulation, and code reuse.
Racket supports OOP through its class system. The class system provides a way to define classes, create objects, and define methods for those objects.
Here's an example of a simple class that defines a rectangle:
#lang racket (define-class rectangle% (init width height) (define width width) (define height height) (define (area) (* width height)))
In this example, we define a class called rectangle%
.
The class has two fields called width
and height
, an init
method that initializes the fields, and a method called area
that calculates the area of the rectangle.
We can create an object of the rectangle%
class like this:
#lang racket (require (class "rectangle.rkt")) (define r (new rectangle% [width 5] [height 10])) (send r area)
This creates an object of the rectangle%
class with a width
of 5
and a height
of 10
.
We can call the area
method on the object using the send
function.
Benefits of OOP
OOP provides several benefits for organizing code:
- Modularity: OOP encourages modularity by breaking code down into smaller, reusable objects.
- Encapsulation: OOP provides encapsulation, which allows objects to hide their internal state and behavior from other objects.
- Code reuse: OOP provides code reuse through inheritance and composition.
- Polymorphism: OOP provides polymorphism, which allows objects to behave differently depending on their type.
Conclusion
In this post, we explored Racket's support for object-oriented programming. We provided an example of a class that defines a rectangle and showed how to create an object of the class and call its methods. We also discussed the benefits of OOP, including modularity, encapsulation, code reuse, and polymorphism. In the next post, we will discuss Racket's support for concurrency and parallelism.