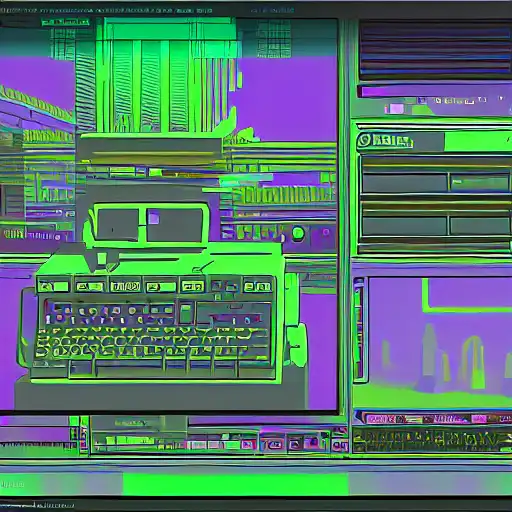
Mar 8th, 2023
Welcome back to our Racket series. In the previous post, we discussed Racket's macro system and its power to extend the language. In this post, we will explore Racket's module system and its benefits for organizing code.
Module System
A module in Racket is a unit of code that provides a namespace for a group of definitions. A module can export definitions that can be used by other modules, and it can import definitions from other modules.
Here's an example of a simple module that defines a function:
#lang racket (module mymodule racket (define (square x) (* x x))) (provide square)
In this example, we define a module called mymodule
.
The module exports a single function called square
that calculates the square of a number.
We can use the square
function like this:
#lang racket (require 'mymodule) (square 5)
This imports the square
function from the mymodule
module and calls it with the argument 5
.
The result is 25
.
Benefits of the Module System
The module system provides several benefits for organizing code:
- Namespacing: Modules provide a namespace for a group of definitions. This makes it easier to avoid naming conflicts and to organize code.
- Encapsulation: Modules can hide implementation details and expose only the definitions that are meant to be used by other modules. This makes it easier to maintain code and to ensure its correctness.
- Reusability: Modules can be reused in multiple projects, making it easier to share code between projects and to maintain a consistent coding style.
- Abstraction: Modules can abstract away complex functionality and provide a simpler interface for other modules to use. This makes it easier to write and maintain code.
Conclusion
In this post, we explored Racket's module system and its benefits for organizing code. We provided an example of a module that exports a function and showed how to import and use the function in another module. We also discussed the benefits of the module system, including namespacing, encapsulation, reusability, and abstraction. In the next post, we will discuss Racket's support for object-oriented programming.