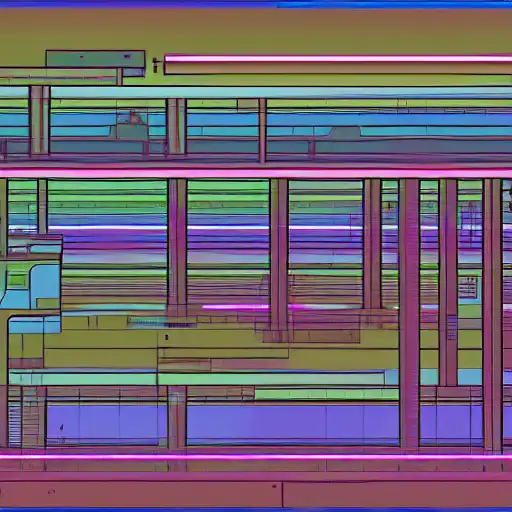
Mar 7th, 2023
Welcome back to our Racket series. In the previous post, we explored Racket's functional programming capabilities, including higher-order functions and closures. In this post, we will discuss Racket's macro system and its power to extend the language.
Macro System
In Racket, a macro is a function that transforms code before it is evaluated. Macros are a powerful feature that allows programmers to extend the language with new constructs and abstractions.
Here's an example of a macro that implements a simple DSL for generating HTML:
#lang racket (define-syntax-rule (html tag attrs content ...) (begin (printf "<~a" ',tag) (for-each (lambda (attr) (printf " ~a=\"~a\"" (car attr) (cdr attr))) ',attrs) (printf ">") ,content (printf "</~a>" ',tag)))
In this example, we define a macro html
that takes a tag name, a list of attributes, and content.
The macro generates HTML code for the given tag with the specified attributes and content.
The macro uses Racket's syntax-quoting and unquoting facilities to generate the code. Syntax-quoting allows us to generate code that looks like Racket code, but is not evaluated immediately. Unquoting allows us to insert expressions into the generated code.
We can use the html
macro like this:
(html h1 '((class . "title")) "Hello, world!")
This generates the following HTML code:
<h1 class="title">Hello, world!</h1>
Extending the Language
The macro system allows Racket programmers to extend the language with new constructs and abstractions. This means that programmers can create domain-specific languages (DSLs) that are tailored to their specific needs.
For example, the html
macro we defined earlier is a simple DSL for generating HTML.
With this macro, we can write HTML code that looks like Racket code.
This makes it easier to write and maintain HTML code, especially for large projects.
Conclusion
In this post, we discussed Racket's macro system and its power to extend the language. We provided an example of a macro that implements a simple DSL for generating HTML. We also discussed how macros can be used to create domain-specific languages that are tailored to specific needs. In the next post, we will explore Racket's module system and its benefits for organizing code.