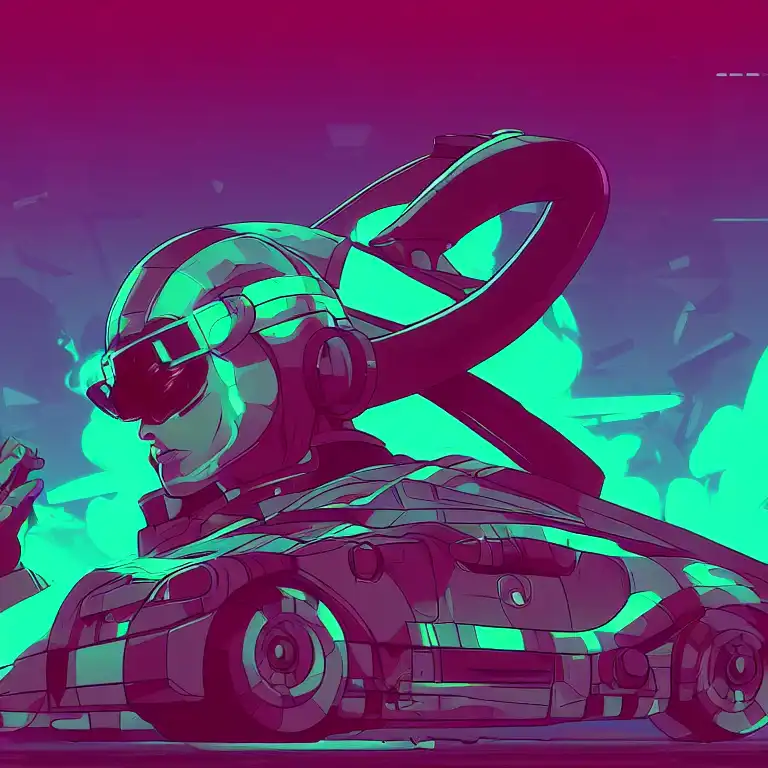
Feb 20th, 2023
OpenCV, or Open Source Computer Vision, is a powerful and widely-used library for image and video processing in Python. It provides a wide range of tools and functions for image and video manipulation, including image filtering, image transformation, feature detection, and more. Whether you're working on a personal project or a professional project, OpenCV is a great choice for image and video processing in Python.
One of the most common tasks in image processing is image filtering. OpenCV provides a wide range of filtering functions, including blur, gaussian blur, median blur, and more.
Another powerful feature of OpenCV is its ability to detect and extract features from images. This can be done using techniques such as edge detection, corner detection, and feature extraction. This is commonly used in computer vision applications such as object detection, facial recognition, and image tracking.
Another important aspect of OpenCV is its ability to process video. It provides a wide range of functions for video capture, playback, and manipulation. This allows developers to create advanced video processing applications such as object tracking, motion detection, and video stabilization.
OpenCV also provides a wide range of functions for image and video display, including window creation and image/video display. This makes it easy to display the results of your image and video processing in a user-friendly manner.
Additionally, OpenCV has a large and active community of developers and researchers who contribute to its development and provide support for users. This means that new features and bug fixes are regularly released, and there is a wealth of resources and tutorials available for learning how to use OpenCV.
For an example of image translation, the following code applies a gaussian blur to an image:
import cv2 # Load an image img = cv2.imread("image.jpg") # Apply a gaussian blur img = cv2.GaussianBlur(img, (5, 5), 0) # Show the image cv2.imshow("Blurred Image", img) cv2.waitKey(0) cv2.destroyAllWindows()
Another common task in image processing is image transformation. OpenCV provides a wide range of image transformation functions, including rotation, scaling, and translation. For example, the following code rotates an image by 45 degrees:
import cv2 import numpy as np # Load an image img = cv2.imread("image.jpg") # Get the image size rows, cols, _ = img.shape # Define the rotation matrix M = cv2.getRotationMatrix2D((cols/2, rows/2), 45, 1) # Rotate the image img = cv2.warpAffine(img, M, (cols, rows)) # Show the image cv2.imshow("Rotated Image", img) cv2.waitKey(0) cv2.destroyAllWindows()
OpenCV also provides a wide range of feature detection functions, such as Harris corner detection, SIFT, and SURF. These functions are useful for image registration, object recognition, and more. For example, the following code detects Harris corners in an image:
import cv2 # Load an image img = cv2.imread("image.jpg") # Convert the image to grayscale gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detect Harris corners gray = np.float32(gray) dst = cv2.cornerHarris(gray, 2, 3, 0.04) # Show the image img[dst>0.01*dst.max()]=[0,0,255] cv2.imshow('Harris Corners', img) cv2.waitKey(0) cv2.destroyAllWindows()
OpenCV also has a module dedicated to video analysis, which contains all the tools needed to process video streams. This includes functions for background subtraction, optical flow analysis, and more.
In conclusion, OpenCV is a powerful and widely-used library for image and video processing in Python. It provides a wide range of tools and functions for image and video manipulation, including image filtering, image transformation, feature detection, and more. Whether you're working on a personal project or a professional project, OpenCV is a great choice for image and video processing