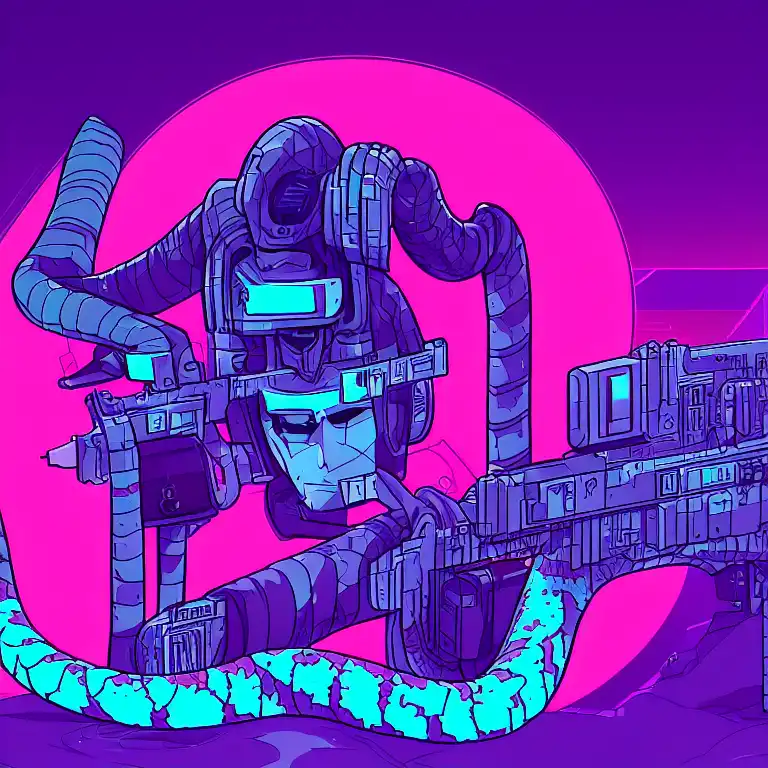
Feb 19th, 2023
Python developers have a lot of options when it comes to working with command-line interfaces (CLIs) and command-line arguments. One popular choice is the Fire library, which provides a simple and easy-to-use API for building CLIs. In this blog post, we'll explore what Fire is and how to use it to create powerful and user-friendly command-line tools.
First, let's start with a brief introduction to Fire. The Fire library is a Python library that makes it easy to build command-line interfaces for Python scripts. It does this by providing a simple and intuitive API for defining commands, handling command-line arguments, and generating usage messages. Fire also supports automatic type coercion and validation of command-line arguments, which makes it easy to write robust and reliable command-line tools.
Now, let's take a look at an example of how to use Fire to create a simple CLI. In this example, we'll create a command-line tool that takes a string and prints it out in uppercase:
import fire def uppercase(string: str): """Prints the given string in uppercase.""" print(string.upper()) if __name__ == "__main__": fire.Fire(uppercase)
This code is pretty straightforward.
We start by importing the fire module, then define a function called uppercase
that takes a single argument, string
, and prints it out in uppercase.
The if __name__ == "__main__":
block at the end of the script ensures that the uppercase
function is only executed when the script is run directly, rather than when it is imported as a module.
Finally, we call fire.Fire(uppercase)
to run our script and make the uppercase
function available as a command-line tool.
When we run this script, we can pass a string to the uppercase
command:
$ python script.py "Hello, World!"
This will output HELLO, WORLD!
Fire also supports defining multiple commands in a single script, using the Fire()
function to run multiple commands.
For example, we can add a new command to our script that reverses a string:
import fire def uppercase(string: str): """Prints the given string in uppercase.""" print(string.upper()) def reverse(string: str): """Prints the given string in reverse order.""" print(string[::-1]) if __name__ == "__main__": fire.Fire()
Now we can use either uppercase
or reverse
command:
$ python script.py uppercase "Hello, World!" $ python script.py reverse "Hello, World!"
This will output HELLO, WORLD!
and !dlroW ,olleH
respectively.
Fire also supports automatic type coercion and validation of command-line arguments.
This means that we can specify the expected types of our command-line arguments, and Fire will automatically convert them to the appropriate Python types.
For example, we can specify that the string
argument should be a string:
def uppercase(string: str): """Prints the given string in uppercase."""
Fire is a powerful command line interface (CLI) library for Python that makes it easy to build and debug command line tools. It is built on top of the argparse module, and provides a simple, intuitive syntax for defining and parsing command line arguments.
One of the key features of Fire is its ability to automatically generate command line interfaces from Python functions and classes. This allows you to quickly and easily build command line tools without having to write any additional code. For example, consider the following simple Python function:
def say_hello(name: str) -> str: return "Hello, " + name
With Fire, we can easily turn this function into a command line tool by simply adding the fire.Fire()
decorator:
import fire @fire.Fire def say_hello(name: str) -> str: return "Hello, " + name
Now, when we run this script from the command line, Fire will automatically generate a command line interface for the say_hello
function, allowing us to call it like this:
$ python script.py say_hello --name "John Doe" Hello, John Doe
In addition to generating command line interfaces for functions and classes, Fire also provides a variety of other features, such as automatic type checking, support for subcommands, and the ability to define default values for arguments.
To illustrate some of these features, let's take a look at a more advanced example. This time, we'll define a class that represents a simple calculator, with methods for performing addition, subtraction, multiplication, and division:
class Calculator: def add(self, a: float, b: float) -> float: return a + b def subtract(self, a: float, b: float) -> float: return a - b def multiply(self, a: float, b: float) -> float: return a * b def divide(self, a: float, b: float) -> float: return a / b
To expose these methods as command line tools, we simply need to add the fire.Fire()
decorator to the class:
import fire @fire.Fire class Calculator: def add(self, a: float, b: float) -> float: return a + b def subtract(self, a: float, b: float) -> float: return a - b def multiply(self, a: float, b: float) -> float: return a * b def divide(self, a: float, b: float) -> float: return a / b
Now, when we run this script from the command line, Fire will generate a command line interface that allows us to call each of the calculator's methods as subcommands, like this:
$ python script.py add --a 2 --b 3 5
$ python script.py subtract --a 2 --b 3 -1
$ python script.py multiply --a 2 --b 3 6
$ python script.py divide --a 6 --b 3 2
In conclusion, Fire is a powerful library for building command-line interfaces in Python. It provides a simple and intuitive way to define and organize commands, and it integrates seamlessly with other Python libraries. With Fire, you can easily create command-line tools that are easy to use and understand for both yourself and others. Whether you're working on a personal project or a professional project, Fire is a great choice for building command-line interfaces.
To learn more about Fire, be sure to check out the official documentation here. Here you'll find more examples, usage tips, and other resources to help you get the most out of this amazing library.
Thank you for reading this post! I hope you found it helpful and informative.