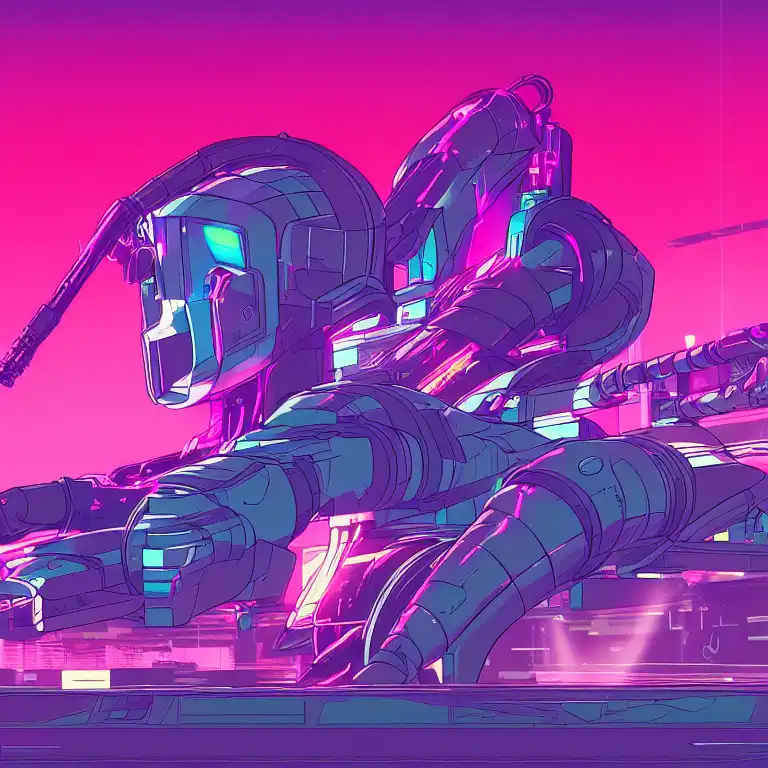
Feb 18th, 2023
SQLAlchemy is a powerful and flexible Object-Relational Mapping (ORM) library for Python. It allows developers to interact with various database systems, such as MySQL, PostgreSQL, and SQLite, using a consistent, Pythonic API. This can greatly simplify the process of working with databases in Python, as it abstracts away many of the low-level details of interacting with the database.
One of the key features of SQLAlchemy is its support for both a "Core" and an "ORM" API. The Core API provides a low-level, SQL-like interface for working with databases, while the ORM API provides a higher-level, object-oriented interface for working with database entities as Python objects. This allows developers to choose the level of abstraction that best suits their needs.
To get started with SQLAlchemy, you will first need to install it using pip: pip install sqlalchemy
Once you have SQLAlchemy installed, you can start by creating a connection to your database. For example, to connect to a SQLite database, you would use the following code:
from sqlalchemy import create_engine engine = create_engine('sqlite:///mydatabase.db')
With the connection established, you can now start interacting with the database. Using the Core API, you can execute raw SQL statements, like so:
from sqlalchemy import text result = engine.execute(text("SELECT * FROM mytable")) print(result.fetchall())
Alternatively, you can use the ORM API to work with database entities as Python objects. First, you will need to define your entities using SQLAlchemy's declarative syntax:
from sqlalchemy.ext.declarative import declarative_base from sqlalchemy import Column, Integer, String Base = declarative_base() class MyEntity(Base): __tablename__ = 'mytable' id = Column(Integer, primary_key=True) name = Column(String)
With your entities defined, you can now create a session to start querying the database:
from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=engine) session = Session() entities = session.query(MyEntity).all() print([entity.name for entity in entities])
These are just a few examples of what you can do with SQLAlchemy. The library provides a wide range of features and options for working with databases, such as support for complex queries, transactions, and connection pooling. Whether you're building a small, data-driven application or a large, enterprise-grade system, SQLAlchemy is a powerful tool that can help you work with databases in Python more effectively.