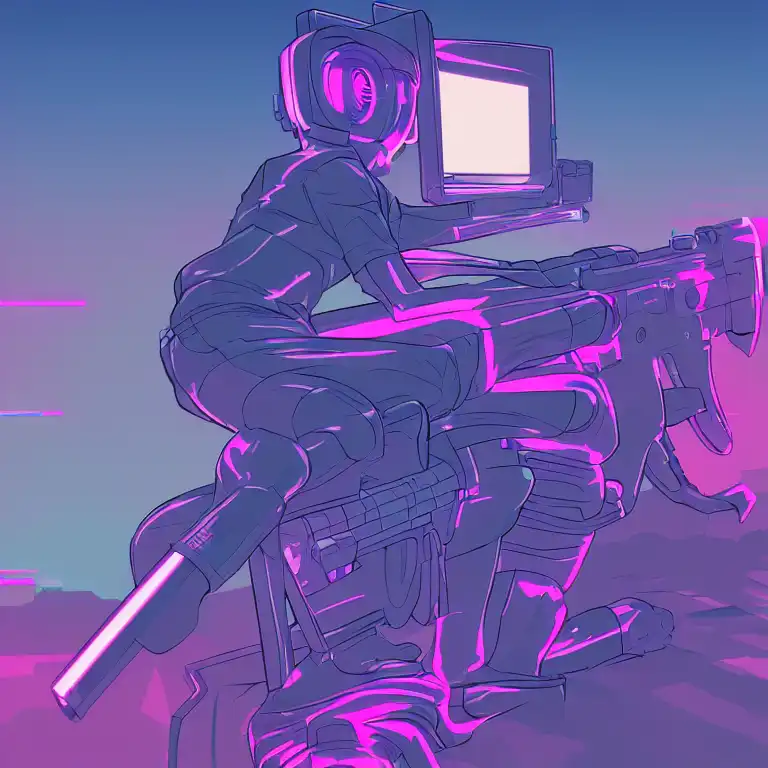
Feb 17th, 2023
PyTorch is an open-source machine learning library for Python that provides a set of tools for building and training neural networks. It is widely used in the field of deep learning and has gained a lot of popularity in recent years due to its ease of use and flexibility.
One of the key features of PyTorch is its dynamic computation graph, which allows for easy modification of the network architecture during training. This allows for a more intuitive and efficient way to build and test models, compared to traditional static computation graphs used in other libraries like TensorFlow.
Another important feature of PyTorch is its support for CUDA, which allows for the use of GPUs to accelerate computations. This is particularly useful for training large and complex models, as it can significantly reduce the time required to train a model.
To get started with PyTorch, you will need to install it first. You can do this by running the following command: pip install torch
Once PyTorch is installed, you can start building and training your own neural networks. A simple example of building a neural network using PyTorch is shown below:
import torch import torch.nn as nn class NeuralNetwork(nn.Module): def __init__(self): super(NeuralNetwork, self).__init__() self.layer1 = nn.Linear(10, 20) self.layer2 = nn.Linear(20, 10) def forward(self, x): x = torch.sigmoid(self.layer1(x)) x = torch.sigmoid(self.layer2(x)) return x model = NeuralNetwork()
In the above example, we define a simple neural network with two fully connected layers.
The nn.Module
is the base class for all neural network modules in PyTorch and nn.Linear
is a fully connected layer.
We also define the forward function, which defines the computation of the network.
Once the model is defined, we can train it using the built-in PyTorch functions. The following code snippet shows an example of how to train a model using PyTorch:
import torch.optim as optim criterion = nn.MSELoss() optimizer = optim.SGD(model.parameters(), lr=0.01) for epoch in range(100): # Forward pass outputs = model(inputs) loss = criterion(outputs, labels) # Backward and optimize optimizer.zero_grad() loss.backward() optimizer.step()
In this example, we use the mean squared error loss function and the stochastic gradient descent optimizer.
The optimizer.zero_grad()
function resets the gradients, and the optimizer.step()
function updates the model's parameters.
PyTorch also offers a variety of pre-trained models, which can be used for transfer learning and fine-tuning.
These models can be easily loaded using the torch.hub
module.
PyTorch is a powerful library that provides a wide range of tools for building and training neural networks. Its dynamic computation graph and CUDA support make it a great choice for deep learning tasks. With its ease of use and flexibility, PyTorch is a great tool for data scientists and machine learning engineers alike.