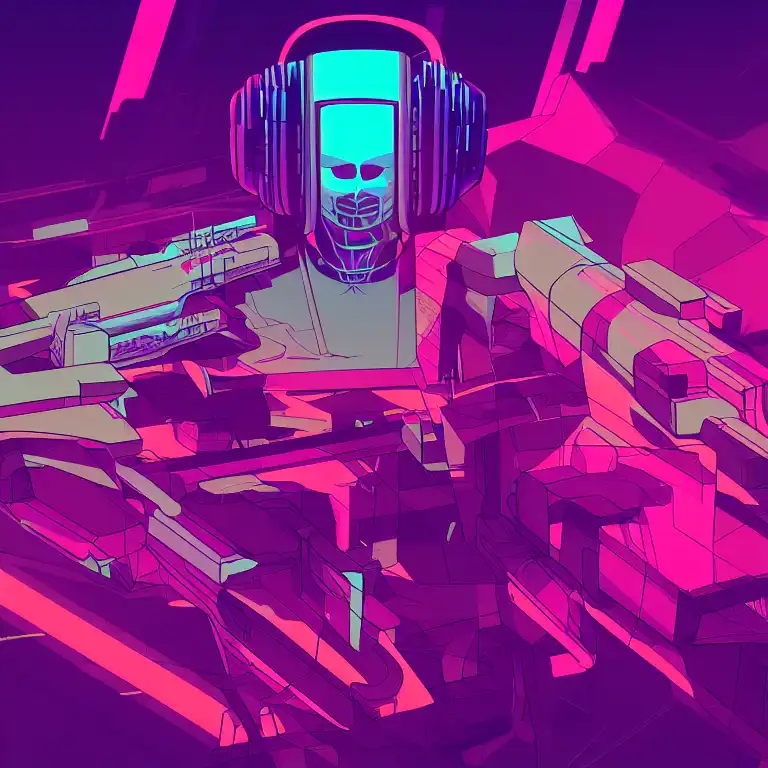
Feb 21st, 2023
Python is a powerful programming language that is widely used in many areas of software development.
One of the most popular libraries that Python developers use is the requests
library.
This library allows you to easily make HTTP requests and handle the responses, making it a powerful tool for web scraping, data collection, and more.
One of the most powerful features of the requests library is its ability to handle different types of requests, including GET, POST, PUT, and DELETE. This allows you to easily interact with web services and APIs, making it a great tool for data collection and manipulation.
Another powerful feature of the requests library is its ability to handle different types of data, including JSON, XML, and HTML. This makes it easy to parse and extract data from web pages, making it a great tool for web scraping.
The requests library also provides a wide range of options for handling different types of authentication and security, such as Basic Auth, OAuth, and SSL/TLS. This makes it easy to work with secure websites and APIs, making it a great tool for a wide range of use cases.
Additionally, the requests library has a large and active community of developers who contribute to its development and provide support for users. This means that new features and bug fixes are regularly released, and there is a wealth of resources and tutorials available for learning how to use the requests library. The library also provides great flexibility to handle different types of requests, including handling cookies, handling redirects, handling timeouts, handling errors, and more.
The requests
library is incredibly simple to use, and you can get started with it in just a few lines of code.
For example, here's how you can make a simple GET request to a website:
import requests response = requests.get("https://www.example.com") print(response.status_code) # 200
This code will make a GET request to the website https://www.example.com
, and then print the status code of the response.
The status code is a three-digit code that indicates the status of the request, such as success or failure.
In this case, we are expecting a status code of 200, which indicates that the request was successful.
You can also make other types of requests, such as POST, PUT, and DELETE. Here's an example of how you can make a POST request:
import requests data = {"name": "John Smith", "email": "john.smith@example.com"} response = requests.post("https://www.example.com/submit", data=data) print(response.status_code) # 200
In this example, we are sending a POST request to the URL https://www.example.com/submit
and passing in a dictionary of data that we want to send to the server.
This is known as the payload of the request.
The server will process this data and return a response.
One of the most powerful features of the requests
library is its ability to handle various types of responses.
You can get the response body in various formats like json, text or binary.
You can also handle cookies and headers.
Here's an example of how you can get the response body as json:
import requests response = requests.get("https://jsonplaceholder.typicode.com/todos/1") if response.status_code == 200: json_data = response.json() print(json_data)
In this example, the response body is json data, the json() method parse the json data and return a python dict object.
In addition to these basic features, the requests
library also provides a number of other features that can help you to make more complex requests.
You can authenticate requests, handle redirects, and even use proxies.
It also allows you to customize headers, cookies, and other settings.
Overall, the requests
library is a powerful and easy-to-use tool that makes it easy to send HTTP requests and handle the responses in Python.
Whether you're working on a web scraping project, a data collection tool, or any other type of application that needs to interact with the web, the requests
library is an essential tool to have in your toolbox.