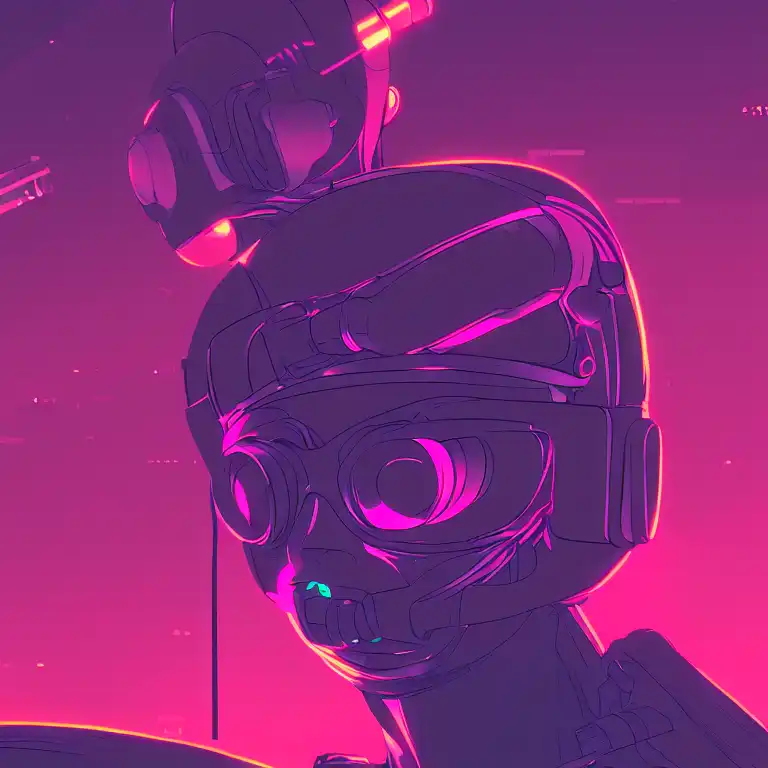
Feb 14th, 2023
Python provides several built-in libraries for mathematical operations, including the math and cmath libraries. These libraries contain a variety of functions for mathematical calculations, including trigonometric, logarithmic, and other common mathematical functions. In this blog post, we will explore the math and cmath libraries in Python and how to use them for various mathematical operations.
The math library is a built-in Python library that contains mathematical functions. Some of the most commonly used functions in the math library include trigonometric functions such as sin, cos, and tan, logarithmic functions such as log and log10, and other mathematical functions such as sqrt, exp, and pow. Here's an example of how to use the math library to calculate the square root of a number:
import math # calculate the square root of 9 result = math.sqrt(9) print(result)
The output of this code will be 3, which is the square root of 9.
The cmath library is similar to the math library, but it is specifically designed for complex numbers. The cmath library provides functions for mathematical operations on complex numbers, such as calculating the square root of a negative number. Here's an example of how to use the cmath library to calculate the square root of -9:
import cmath # calculate the square root of -9 result = cmath.sqrt(-9) print(result)
The output of this code will be (0+3j), which is the square root of -9 in complex number form.
In addition to these common mathematical functions, the math and cmath libraries also provide other useful functions such as the ability to calculate the factorial of a number, the greatest common denominator, and the least common multiple.
import math #calculating factorial result = math.factorial(5) print(result)
The output of this code will be 120, which is the factorial of 5 (5*4*3*2*1)
import math #calculating GCD result = math.gcd(20, 8) print(result)
The output of this code will be 4, which is the greatest common denominator of 20 and 8
In conclusion, the math and cmath libraries in Python provide a wide range of mathematical functions that can be used for various mathematical operations. Whether you're working with basic arithmetic or more complex calculations involving complex numbers, these libraries can help make your code more efficient and easy to read. By using the math and cmath libraries, you can perform calculations with less code and less error-prone. So, it is always good to have a good understanding of these libraries in order to use them efficiently in our projects.