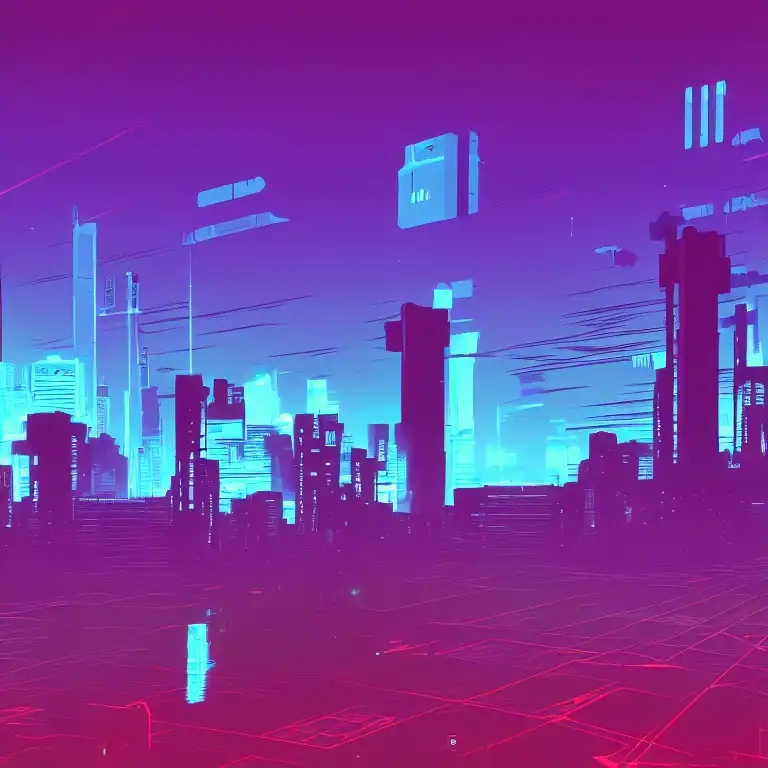
Feb 13th, 2023
The hashlib
library in Python is a powerful tool for creating and working with cryptographic hash functions.
A hash function is a one-way function that takes an input (or "message") and returns a fixed-size string of characters, which is a "digest" that serves as a unique representation of the input.
The most common use of hash functions is in the creation of digital signatures and the validation of data integrity.
One of the key features of the hashlib
library is that it provides a simple and consistent interface for working with a wide variety of hash algorithms.
Some of the most commonly used algorithms include SHA-1, SHA-256, and SHA-512, which are all part of the Secure Hash Algorithm (SHA) family.
Here is an example of how to use the hashlib
library to create a hash of a message using the SHA-256 algorithm:
import hashlib message = "Hello, World!" # Create a new SHA-256 hash object hash_object = hashlib.sha256() # Update the hash object with the bytes of the message hash_object.update(message.encode()) # Get the hexadecimal representation of the hash hash_hex = hash_object.hexdigest() print(hash_hex)
In this example, we first import the hashlib
library and define a message to be hashed.
Next, we create a new SHA-256 hash object using the hashlib.sha256()
function.
We then update the hash object with the bytes of the message using the update()
method.
Finally, we use the hexdigest()
method to get the hexadecimal representation of the hash.
Another feature of the hashlib
library is the ability to create a hash from a file.
Here's an example of how to create a hash of a file using the SHA-256 algorithm:
import hashlib with open("example.txt", "rb") as f: # Create a new SHA-256 hash object hash_object = hashlib.sha256() # Read the file in chunks while True: data = f.read(4096) if not data: break hash_object.update(data) # Get the hexadecimal representation of the hash hash_hex = hash_object.hexdigest() print(hash_hex)
In this example, we open the file "example.txt" in binary mode using the open()
function.
Then we create a new SHA-256 hash object.
We read the file in chunks using the read()
method, updating the hash object with each chunk of data.
Finally, we use the hexdigest()
method to get the hexadecimal representation of the hash.
In conclusion, the hashlib
library in Python is a powerful tool for working with cryptographic hash functions.
It provides a simple and consistent interface for working with a wide variety of hash algorithms, and it's easy to create a hash from a message or a file.
It's an important tool for data integrity, digital signatures and other cryptographic operations.