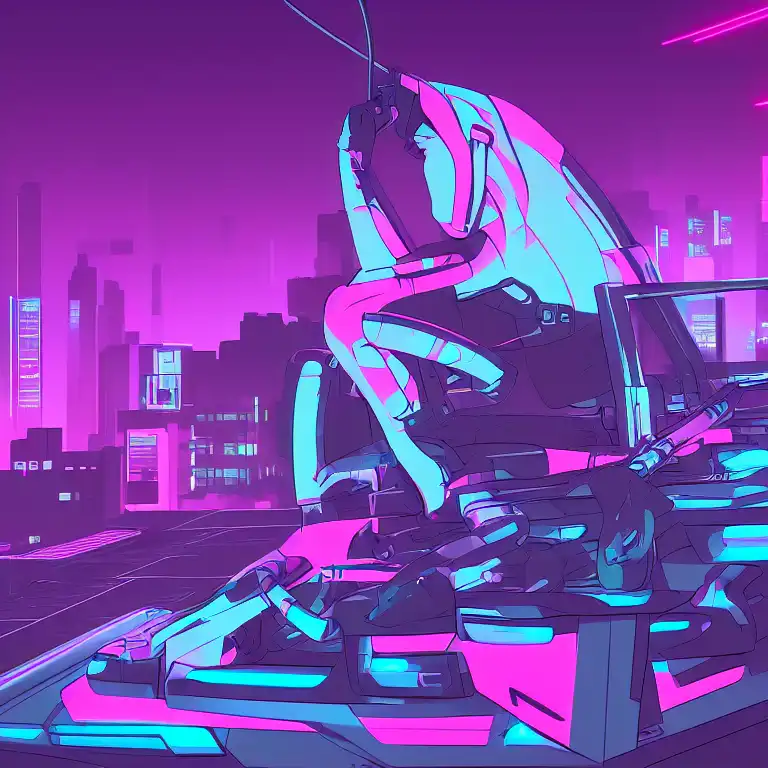
Feb 15th, 2023
Python is a versatile and powerful programming language, and its libraries make it even more so. One of the most popular libraries for creating games and other interactive applications is Pygame. Pygame is a set of Python modules designed for writing video games. It is highly portable, and runs on almost every platform, including Windows, MacOS, and Linux.
Pygame is built on top of the SDL library, which provides low-level access to audio, keyboard, mouse, and display functions. This means that Pygame applications can be written in pure Python, without the need for any C or C++ code. Pygame provides a simple and easy-to-use API, making it ideal for beginners and experts alike.
To get started with Pygame, we first need to install it. This can be done using pip: pip install pygame
Once we have Pygame installed, we can start writing our first game. Here is a simple example of a Pygame program that creates a window and displays a moving rectangle:
import pygame # Initialize Pygame pygame.init() # Create a window screen = pygame.display.set_mode((400, 300)) pygame.display.set_caption("My First Pygame Program") # Run the game loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Clear the screen screen.fill((0, 0, 0)) # Draw a rectangle pygame.draw.rect(screen, (255, 0, 0), (20, 20, 60, 40)) # Update the display pygame.display.flip() # Quit Pygame pygame.quit()
In this example, we first import the Pygame library, then initialize it using pygame.init()
.
Next, we create a window using pygame.display.set_mode((400, 300))
and give it a title using pygame.display.set_caption("My First Pygame Program")
.
The game loop is the heart of any Pygame program. It repeatedly handles events and updates the display. In this example, the game loop checks for the QUIT event, which is generated when the user closes the window. If the QUIT event is detected, the loop is terminated and the program exits.
Inside the game loop, we first clear the screen using screen.fill((0, 0, 0))
and then draw a rectangle on it using pygame.draw.rect(screen, (255, 0, 0), (20, 20, 60, 40))
.
Finally, we update the display using pygame.display.flip()
.
Pygame also provides many other features, such as image and sound loading, input handling, and collision detection. These features can be used to create more advanced games, such as platformers, shooters, and puzzle games.
Overall, Pygame is a powerful and easy-to-use library for creating games and other interactive applications in Python. It provides a simple and intuitive API, and its wide range of features makes it suitable for beginners and experts alike. With Pygame, you can bring your game ideas to life and create engaging and fun applications.