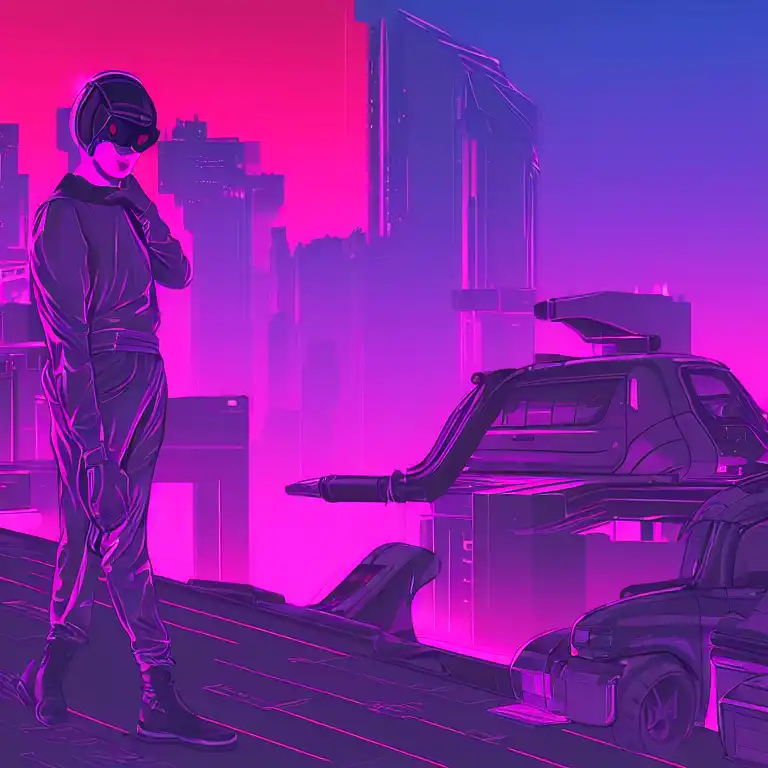
Feb 9th, 2023
Iterators and iterables are fundamental concepts in Python that allow you to work with collections of data in a clean and efficient manner. In this post, we'll take a deep dive into iterators and iterables, explore how they work, and see how you can use them to simplify and streamline your Python code.
An iterable is an object that can be iterated (looped) upon.
It defines a method called iter()
which returns an iterator object.
Examples of iterable objects include lists, tuples, strings, and dictionaries.
An iterator is an object that is used to iterate over an iterable.
It defines two methods: next()
and iter()
. The next()
method returns the next item in the iterable, while the iter()
method returns the iterator object itself.
This allows the iterator to be used in a for loop.
Here's an example of how you can use a for loop to iterate over a list:
numbers = [1, 2, 3, 4, 5] for number in numbers: print(number)
In this example, the for loop is used to iterate over the list "numbers". On each iteration, the variable "number" is assigned the next value in the list. This process continues until all the values in the list have been processed.
An iterator is an object that is used to iterate over an iterable object.
It defines two methods, iter()
and next()
.
The iter()
method returns the iterator object itself.
The next()
method returns the next value from the iterable object.
If there are no more items to return, it should raise StopIteration
.
Here's an example of how you can use the iterator protocol to manually iterate over a list:
numbers = [1, 2, 3, 4, 5] iterator = iter(numbers) print(next(iterator)) # 1 print(next(iterator)) # 2 print(next(iterator)) # 3 print(next(iterator)) # 4 print(next(iterator)) # 5
In this example, the iter(numbers)
function returns an iterator object that can be used to iterate over the list "numbers".
The next(iterator)
function is used to retrieve the next value from the iterator.
As you can see, this process is equivalent to the for loop example above, but with more manual control over the iteration process.
Another example of Iterator is the built-in enumerate()
function, it takes an iterable and returns an iterator that produces tuples containing the index and the corresponding item.
words = ["hello", "world"] for index, word in enumerate(words): print(index, word)
In this example, the enumerate()
function is used to iterate over the list "words" and returns an iterator that produces tuples containing the index and the corresponding item.
In addition to the built-in iterable and iterator objects, you can also create your own.
To create your own iterable object, you need to define the __iter__()
method which should return an iterator object.
To create your own iterator object, you need to define the __iter__()
and __next__()
methods.
Here's an example of how you can create a custom iterator that generates the Fibonacci sequence:
class Fibonacci: def __init__(self, max_value): self.max_value = max_value self.a = 0 self.b = 1 def __iter__(self): return self def __next__(self): fib = self.a if fib > self.max_value: raise StopIteration self.a, self.b = self.b, self.a + self.b return fib fibonacci = Fibonacci(20) for n in fibonacci: print(n)
In this example, we have defined a class called Fibonacci
that has an __iter__()
method that returns an iterator object and a __next__()
method that calculates the next Fibonacci number.
The __init__()
method sets the maximum value for the sequence and initializes the first two values of the sequence.
In the for loop, we are able to iterate over the fibonacci object as it is an iterable, and the for loop will call the next()
method of the iterator returned by the __iter__
method.
In conclusion, iterators and iterables are powerful and versatile features of Python that allow you to work with collections of data in a clean and efficient manner. By understanding how they work, you can use them to simplify and streamline your Python code, making it more readable and maintainable. Whether you're working with built-in iterable and iterator objects or creating your own, the possibilities are endless.