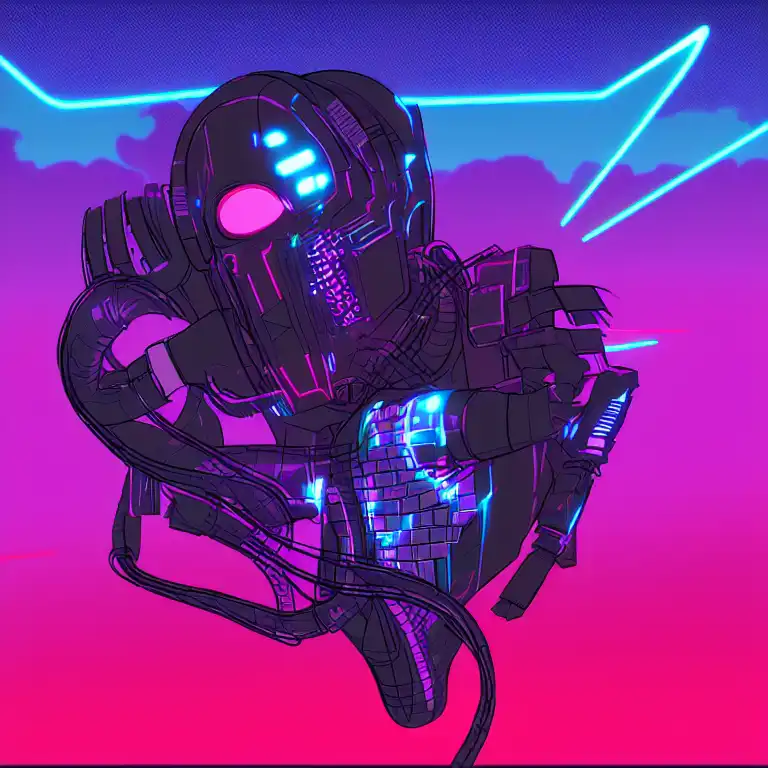
Feb 10th, 2023
Matplotlib is a powerful data visualization library in Python that allows you to create a wide variety of plots and charts. Whether you're working with numerical data or categorical data, Matplotlib has a variety of tools and options to help you create high-quality visualizations that communicate your findings effectively. In this blog post, we will explore some of the key features of Matplotlib and how to use them to create beautiful and informative plots.
One of the most basic and widely used plots in Matplotlib is the line plot.
Line plots are used to display the relationship between two numerical variables, such as time and temperature.
To create a line plot in Matplotlib, you will first need to import the pyplot
module from the matplotlib
library. Then, you can use the plot()
function to create a line plot of your data.
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [5, 7, 2, 8, 4] plt.plot(x, y) plt.show()
In this example, we have created a line plot of x and y values. The x
variable represents the x-axis and the y
variable represents the y-axis. The show()
function is used to display the plot on the screen.
Another popular plot in Matplotlib is the scatter plot, which is used to display the relationship between two numerical variables.
Scatter plots are useful for visualizing the distribution of data and identifying patterns and outliers.
To create a scatter plot in Matplotlib, you can use the scatter()
function.
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [5, 7, 2, 8, 4] plt.scatter(x, y) plt.show()
In this example, we have created a scatter plot of x and y values.
The x
variable represents the x-axis and the y
variable represents the y-axis.
The show()
function is used to display the plot on the screen.
Another important aspect of Matplotlib is the ability to customize plots with different styles and options.
For example, you can change the color, line style, and marker style of your plot using the color
, linestyle
, and marker
parameters.
import matplotlib.pyplot as plt x = [1, 2, 3, 4, 5] y = [5, 7, 2, 8, 4] plt.plot(x, y, color='green', linestyle='dashed', marker='o') plt.show()
In this example, we have customized the line plot by setting the color to green, the line style to dashed and the marker to circle.
Matplotlib also provides a variety of options for creating bar charts, pie charts, histograms, and other types of plots. You can also customize the appearance of your plots by adjusting the axis labels, tick marks, and other elements of the plot.
In conclusion, Matplotlib is a powerful and versatile library for creating data visualizations in Python. With its wide range of tools and options, you can create a variety of plots and charts that effectively communicate your findings. Whether you're working with numerical data or categorical data, Matplotlib has the tools you need to create high-quality visualizations that are both beautiful and informative. With its easy-to-use API, you can quickly and easily create plots and charts that are tailored to your specific needs.
In addition to its basic plotting capabilities, Matplotlib also provides advanced features such as 3D plotting, animation, and interactive plotting. This makes it a great choice for creating professional-quality visualizations for data science, engineering, and scientific research.
If you're new to Matplotlib, there are plenty of resources available to help you get started. The Matplotlib documentation provides a wealth of information on the library's features and capabilities, and there are many tutorials and examples available online. Additionally, the Matplotlib community is very active and there are many resources and support available to help you with any questions or issues that you may encounter.
In conclusion, Matplotlib is an essential tool for any Python developer who needs to create data visualizations. It is a powerful, versatile, and easy-to-use library that provides a wide range of tools and options for creating high-quality plots and charts. Whether you're a beginner or an experienced developer, Matplotlib is a library that you should definitely consider when working with data in Python.