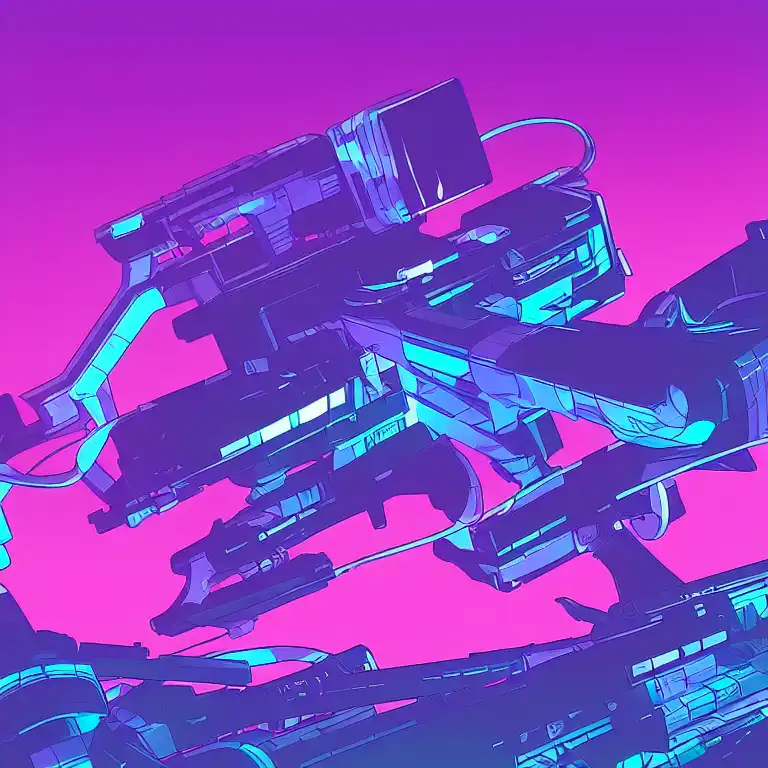
Feb 8th, 2023
Context managers are a powerful feature in Python that allow you to manage resources, such as files or database connections, in a safe and efficient manner.
A context manager is an object that defines the methods enter()
and exit()
.
These methods are used to set up and tear down a context for a block of code.
In this post, we'll take a deep dive into context managers and explore how they can be used to simplify and streamline your Python code.
The most common use of context managers is to work with files.
The built-in open()
function in Python is a context manager.
Context managers can also be used to handle other types of resources. For example, you can use a context manager to manage a database connection, ensuring that the connection is properly closed when you're finished with it. This is especially useful when working with databases that have a limited number of connections available.
Another advantage of context managers is that they make it easy to handle errors and exceptions.
With a context manager, you can use the try-finally block to ensure that the exit()
method is called, even if an exception is raised within the block of code.
This makes it easy to clean up resources, even in the event of an error.
You can also create your own context managers by using the contextlib library. The contextlib library provides a decorator called contextmanager that can be used to define a context manager. You can also use this library to create context managers using generators.
Here's an example of how you can use a context manager to read a file:
with open('example.txt', 'r') as file: print(file.read())
In this example, the with statement is used to create a context for the block of code that follows.
The open()
function is called with the file name and the mode, and it returns a file object that is assigned to the variable "file".
The block of code inside the with statement can then use the file object to read the contents of the file.
When the block of code finishes executing, the context is closed, and the file object's exit()
method is called, which automatically closes the file.
Another common use of context managers is to work with database connections. Here's an example of how you can use a context manager to connect to a database and execute a query:
import sqlite3 with sqlite3.connect('example.db') as connection: cursor = connection.cursor() cursor.execute('SELECT * FROM example_table') results = cursor.fetchall() print(results)
In this example, the with statement is used to create a context for the block of code that follows.
The sqlite3.connect()
function is called with the database name and it returns a connection object that is assigned to the variable "connection".
The block of code inside the with statement can then use the connection object to execute queries and fetch results.
When the block of code finishes executing, the context is closed, and the connection object's exit()
method is called, which automatically closes the connection.
You can also create your own context manager by creating a class that defines the enter()
and exit()
methods, and then using the contextlib.contextmanager decorator to create a generator-based context manager.
Here's an example of how you can create a context manager that times a block of code:
import time from contextlib import contextmanager @contextmanager def timer(): start_time = time.time() yield end_time = time.time() print('Elapsed time:', end_time - start_time) with timer(): # some code to be timed time.sleep(1)
In this example, the timer()
function is decorated with the @contextmanager
decorator.
Inside the function, the start_time
is recorded, and the yield statement is used to indicate the start of the context block.
The code inside the with statement can then execute.
After the code inside the with statement is finished, the end_time
is recorded and the difference is printed.
In conclusion, context managers are a powerful feature in Python that allow you to manage resources in a safe and efficient manner. They are particularly useful when working with files, database connections, and other resources that need to be set up and torn down on a regular basis. By using context managers, you can ensure that your resources are properly closed, even in the event of an exception. Additionally, context managers can help you to simplify your code by eliminating the need for explicit try-finally blocks, making your code more readable and maintainable. To take full advantage of context managers, it's important to understand how they work and when they should be used. With a good understanding of context managers, you'll be able to write more robust and efficient code in Python.