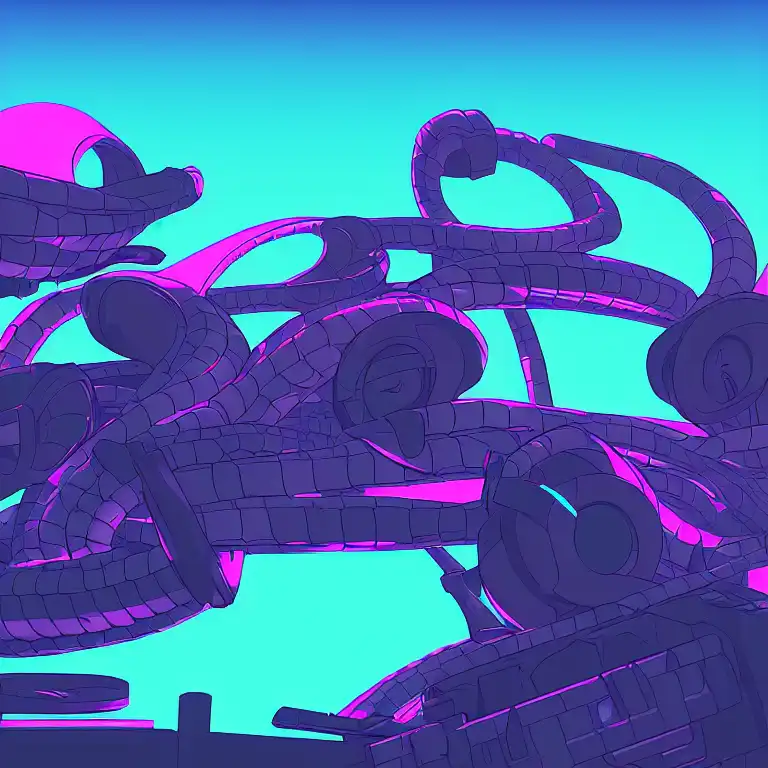
Feb 7th, 2023
Python is a powerful programming language that provides a wide range of features for working with data. One of the most powerful tools in Python is the set of built-in functional programming tools, which include the lambda operator, filter, reduce, and map functions. In this post, we'll take a deep dive into these features and explore how they can be used to simplify and streamline your Python code.
First up, let's take a look at the lambda operator. The lambda operator is a way to create small, anonymous functions in Python. These functions can be used to perform simple operations, such as mathematical calculations or string manipulation. Here's an example of a lambda function that takes a single argument and returns its square:
square = lambda x: x * x print(square(5)) # 25
The lambda operator is often used in conjunction with other functional programming tools, such as filter and map.
The filter function is used to filter a list of items based on a given condition. For example, we can use the filter function to find all the even numbers in a list:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] evens = filter(lambda x: x % 2 == 0, numbers) print(list(evens)) # [2, 4, 6, 8, 10]
In this example, the filter function is passed two arguments: a lambda function that checks if a number is even, and a list of numbers. The filter function applies the lambda function to each element of the list and returns a new list containing only the elements that satisfy the condition.
Next, let's take a look at the reduce function. The reduce function is used to combine all the elements of a list into a single value. For example, we can use the reduce function to find the product of all the numbers in a list:
from functools import reduce numbers = [1, 2, 3, 4, 5] product = reduce(lambda x, y: x * y, numbers) print(product) # 120
In this example, the reduce function is passed two arguments: a lambda function that multiplies two numbers, and a list of numbers. The reduce function applies the lambda function to the first two elements of the list, then to the result and the next element, and so on, until it has combined all the elements of the list into a single value.
Finally, let's take a look at the map function. The map function is used to apply a function to all the elements of a list. For example, we can use the map function to square all the numbers in a list:
numbers = [1, 2, 3, 4, 5] squared = map(lambda x: x * x, numbers) print(list(squared)) # [1, 4, 9, 16, 25]
In this example, the map function is passed two arguments: a lambda function that squares a number, and a list of numbers. The map function applies the lambda function to each element of the list and returns a new list containing the results.
In conclusion, the lambda operator, filter, reduce, and map functions are powerful tools in Python that allow you to write more concise and expressive code. They are particularly useful when working with large sets of data, and can help you to avoid writing complex and error-prone loops. With a good understanding of these functional programming tools, you'll be able to take your Python skills to the next level and tackle more complex problems with ease. It is always a good idea to practice using these tools in a variety of different situations to become more familiar with their capabilities and limitations. Additionally, it's important to keep in mind that while these functional programming tools can be very powerful, they should be used judiciously and in conjunction with other programming techniques. By combining the use of functional programming tools with other techniques such as object-oriented programming, you can create more robust, efficient and maintainable code. Overall, the lambda operator, filter, reduce and map functions are a valuable addition to any Python developer's toolkit and are definitely worth taking the time to learn.