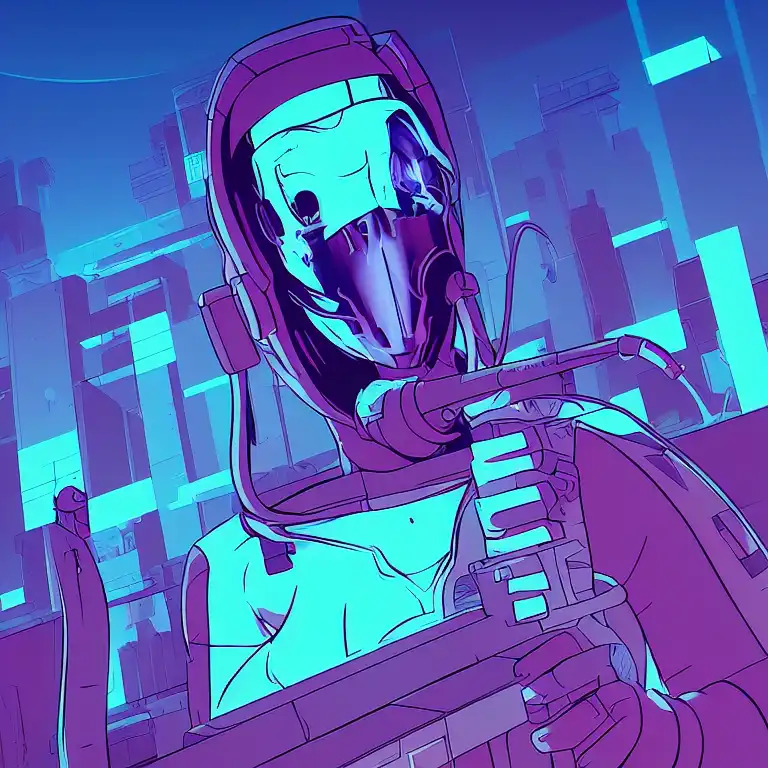
Feb 6th, 2023
The pickle library for Python is a powerful tool for serializing and deserializing Python objects. This means that you can convert an object in memory to a byte stream, which can then be stored to a file or sent over a network. Later on, this byte stream can be converted back into an object, allowing you to easily save and load data in your Python applications.
One of the most basic use cases for the pickle library is to save a Python object to a file. In addition to saving objects to a file, the pickle library can also be used to send objects over a network. This can be useful in situations where you need to share data between different machines or processes. For example, you could use the pickle library to send a complex object from one server to another, or to send data from a Python script to a web application.
Another useful feature of the pickle library is the ability to customize the serialization process. By default, pickle will serialize all of the attributes of an object, but you can also specify which attributes should be included or excluded. Additionally, you can use the pickle library to specify custom logic for serializing or deserializing specific types of objects.
It's important to note that while the pickle library is a powerful tool, it should be used with caution. Because pickle allows you to deserialize arbitrary byte streams, it can also be used to execute malicious code. Therefore it is important to only unpickle data from trusted sources.
Additionally, the pickle library is specific to Python. If you need to share data between different programming languages, you may want to consider using a more widely-supported serialization format like JSON or XML.
Here's an example of how to use pickle to save a list of integers to a file:
import pickle numbers = [1, 2, 3, 4, 5] with open("numbers.pickle", "wb") as file: pickle.dump(numbers, file)
This code creates a list of integers and uses the pickle.dump()
function to write the list to a file called "numbers.pickle" in binary mode.
The pickle.dump()
function takes two arguments: the object to be serialized and the file object to which the object should be written.
To load the data back from the file, you can use the pickle.load()
function.
Here's an example:
with open("numbers.pickle", "rb") as file: numbers = pickle.load(file) print(numbers) # [1, 2, 3, 4, 5]
The pickle.load()
function reads the bytes from the file and converts them back into the original Python object.
In this case, the original list of integers.
Pickling can also be used to send data over the network, for example using sockets. Here's an example of using pickling to send an object over a socket:
import socket import pickle def send_obj(sock, obj): data = pickle.dumps(obj) sock.sendall(data) def recv_obj(sock): data = b'' while True: part = sock.recv(1024) data += part if len(part) < 1024: break return pickle.loads(data) sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.connect(('localhost', 1234)) obj = {'a':1,'b':2,'c':3} send_obj(sock, obj) received_obj = recv_obj(sock) print(received_obj)
This example defines two functions, send_obj()
and send_obj()
, that can be used to send and receive objects over a socket.
The send_obj()
function uses the pickle.dumps()
function to convert the object to a byte stream and then sends it over the socket using the sock.sendall()
method.
The send_obj()
function receives bytes from the socket and then uses the pickle.loads()
function to convert the byte stream back into an object.
It's important to note that pickling is not safe, it can be manipulated by malicious actors. The pickle module is not intended to be secure against erroneous or maliciously constructed data. Never unpickle data received from an untrusted or unauthenticated source.
In conclusion, the pickle library in Python is a powerful tool for serializing and deserializing Python objects. It allows you to easily save and load data in your Python applications, as well as send data over a network. However, it is important to keep in mind that pickling is not safe, and it should never be used with untrusted or unauthenticated data. Instead, you should consider using more secure alternatives such as JSON or msgpack. Overall, pickling is a useful tool to have in your toolbox, but it's important to use it with caution.
In this post, we've covered the basics of using the pickle library in Python, including how to save and load data to and from a file, and how to send and receive data over a network using sockets. I hope this article has been helpful in understanding the pickle library and its uses.