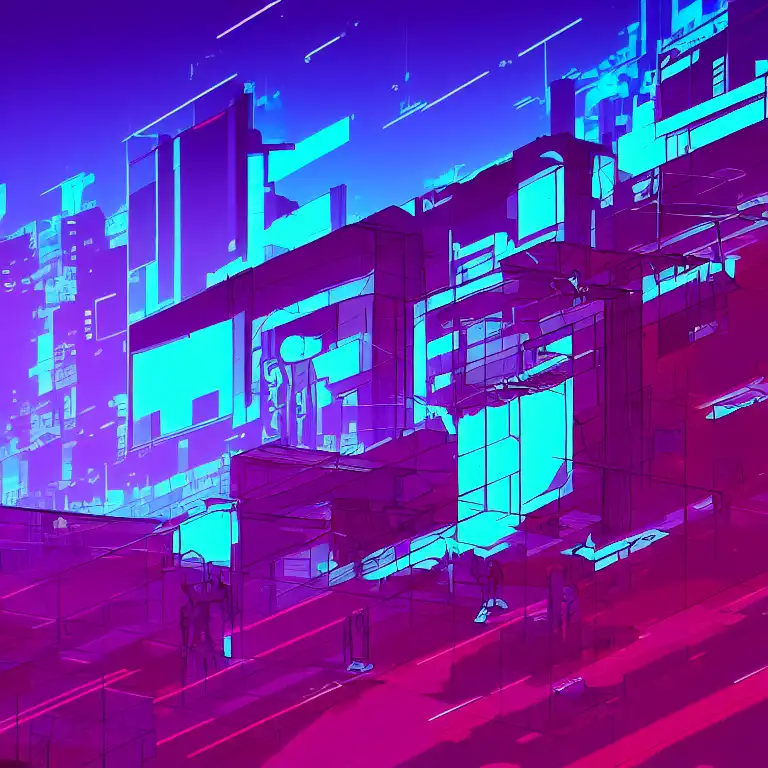
Feb 5th, 2023
PyQt5 is a powerful library for creating desktop applications in Python. It is a set of Python bindings for the Qt libraries, which provides a comprehensive set of widgets and other tools for building user interfaces. In this post, we'll explore the basics of PyQt5 and how to use it to create a simple application.
To get started with PyQt5, you'll need to install the library. You can do this using pip: pip install PyQt5
Once you have PyQt5 installed, you can start creating your application.
The first step is to import the necessary modules from the library: from PyQt5.QtWidgets import QApplication, QLabel
The QApplication
class is the main event loop for a PyQt5 application, and the QLabel
class is a widget for displaying text.
Next, we'll create an instance of the QApplication
class and a label widget:
app = QApplication([]) label = QLabel("Hello, PyQt5!")
The QApplication
class takes a list of command line arguments as an argument.
In this case, we're passing an empty list.
We can then show the label on the screen by calling the show()
method:
label.show()
Finally, we'll start the event loop by calling the exec_()
method of the QApplication
class:
app.exec_()
This will keep the application running until the user closes the window.
The above example is a basic PyQt5 application, it creates a window with a label that displays text "Hello, PyQt5!". But PyQt5 provides many more widgets such as Buttons, LineEdit, ComboBox, ListView, etc.
from PyQt5.QtWidgets import QApplication, QLabel, QLineEdit, QPushButton app = QApplication([]) label = QLabel("Please enter your name:") line_edit = QLineEdit() button = QPushButton("Submit") label.show() line_edit.show() button.show() app.exec_()
In this example, we've added a QLineEdit and a QPushButton to the application, which allows the user to enter their name and submit it.
PyQt5 also provides a powerful layout system that allows you to control the position and size of widgets.
The basic layout classes are QHBoxLayout
, QVBoxLayout
, and QGridLayout
.
These classes allow you to arrange widgets in a horizontal, vertical, or grid pattern.
from PyQt5.QtWidgets import QApplication, QLabel, QLineEdit, QPushButton, QVBoxLayout app = QApplication([]) label = QLabel("Please enter your name:") line_edit = QLineEdit() button = QPushButton("Submit") layout = QVBoxLayout() layout.addWidget(label) layout.addWidget(line_edit) layout.addWidget(button) widget = QWidget() widget.setLayout(layout) widget.show() app.exec_()
In this example, we've added the QVBoxLayout
to the application which will arrange the widgets vertically.
The addWidget()
method is used to add the label, line edit, and button to the layout.
We then create a QWidget
and set the layout to be the QVBoxLayout
that we created. Finally, we show the widget and start the event loop.
PyQt5 also allows you to handle signals and slots, which is a mechanism for communication between objects. Signals are emitted by widgets when an event occurs, such as a button being clicked. Slots are methods that are called when a signal is emitted.
from PyQt5.QtWidgets import QApplication, QLabel, QLineEdit, QPushButton, QVBoxLayout app = QApplication([]) label = QLabel("Please enter your name:") line_edit = QLineEdit() button = QPushButton("Submit") def on_button_clicked(): name = line_edit.text() label.setText("Hello, " + name + "!") button.clicked.connect(on_button_clicked) layout = QVBoxLayout() layout.addWidget(label) layout.addWidget(line_edit) layout.addWidget(button) widget = QWidget() widget.setLayout(layout) widget.show() app.exec_()
In this example, we've added a new method called on_button_clicked()
which is connected to the clicked
signal of the button.
When the button is clicked, the on_button_clicked()
method is called, which retrieves the text from the line edit and sets it as the text of the label.
In this post, we've covered the basics of PyQt5, including how to create a simple application, use widgets, layouts, and signals and slots. PyQt5 is a powerful library that allows you to create rich, interactive desktop applications in Python. With its comprehensive set of widgets, powerful layout system, and support for signals and slots, you can build almost any kind of application you can imagine. I hope this article has helped you to get started with PyQt5, and that you'll be able to use it to create your own amazing applications!