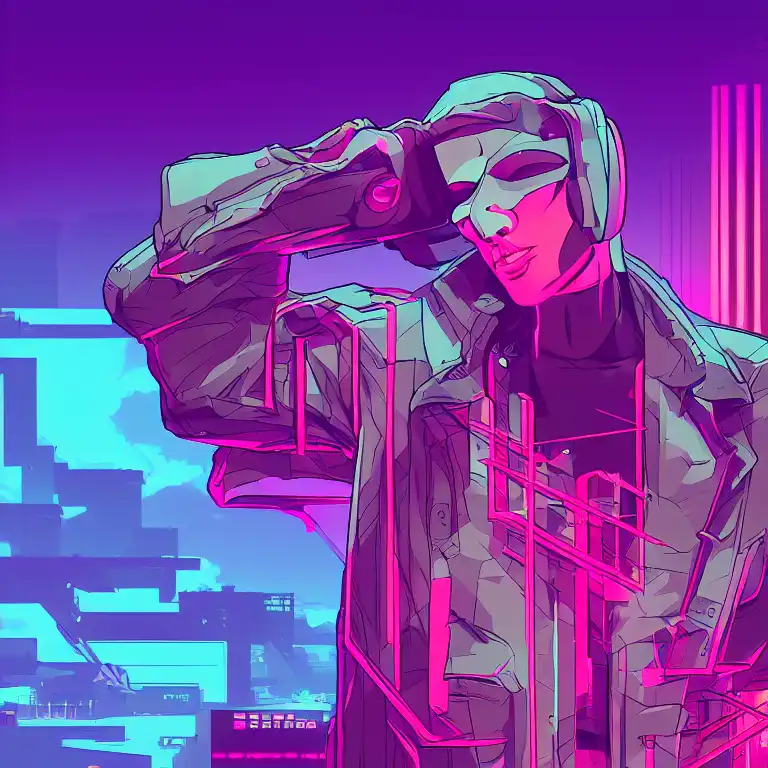
Feb 4th, 2023
Decorators are a powerful feature in Python that allow you to modify the behavior of a function or class without changing its source code. They are often used to add functionality, such as logging or caching, to existing code. In this post, we'll explore the basics of decorators and how to use them in your own projects.
A decorator is simply a function that takes another function as an argument and returns a new function that modifies the behavior of the original function. The new function is often referred to as the "decorated" function. Here's a simple example of a decorator that logs the arguments and return value of a function:
When using decorators, it is important to keep in mind that the original function remains unchanged and only the behavior of the function is modified. This means that the original function can still be used as it was originally intended, without any of the added functionality provided by the decorator.
Decorators can also be used to add functionality to classes, in a similar way to how they are used to modify functions. For example, a decorator can be used to add logging to all of the methods within a class, or to add caching to a specific method.
Additionally, decorators can also be chained together to create more complex functionality. For example, you can use multiple decorators to add logging and caching to a function or class, or you can use a decorator to add a specific feature and then chain it with another decorator to add an additional feature.
It is important to note that decorators are not limited to Python and can be used in other programming languages as well. However, the syntax and usage may differ. With the understanding of decorators, you can use this powerful feature to make your code more efficient, readable, and maintainable. Here's a simple example of a decorator that logs the arguments and return value of a function:
def log_args_and_return(func): def decorated(*args, **kwargs): print(f"Calling {func.__name__} with args {args} and kwargs {kwargs}") result = func(*args, **kwargs) print(f"{func.__name__} returned {result}") return result return decorated @log_args_and_return def add(a, b): return a + b add(1, 2) # Output: # Calling add with args (1, 2) and kwargs {} # add returned 3
In this example, the log_args_and_return
function is our decorator.
It takes a function func
as an argument and returns a new function decorated
that calls the original function and logs the arguments and return value.
We then use the @ symbol to "decorate" the add
function with the log_args_and_return
decorator.
You can also apply multiple decorators to a single function by stacking them on top of each other, like so:
def multiply(a, b): return a * b @log_args_and_return @another_decorator def multiply(a, b): return a * b
In this example, multiply
is first decorated with log_args_and_return
and then with another_decorator
Decorators can also be applied to class methods. In this case, the decorator function takes the class method as an argument and returns a new method that modifies the behavior of the original method. Here's an example of a decorator that logs the execution time of a method:
import time def log_execution_time(method): def decorated(self, *args, **kwargs): start_time = time.time() result = method(self, *args, **kwargs) end_time = time.time() print(f"{method.__name__} took {end_time - start_time} seconds to execute") return result return decorated class MyClass: def __init__(self): pass @log_execution_time def my_method(self): time.sleep(1) my_obj = MyClass() my_obj.my_method() # Output: # my_method took 1.000377893447876 seconds to execute
In this example, the log_execution_time
decorator is applied to the log_execution_time
method of the MyClass
In conclusion, decorators are a useful tool for modifying the behavior of functions and classes in Python. They allow you to add functionality to existing code without changing the source code, making it easier to maintain and test. By using decorators, you can keep your code clean and organized, and make it more reusable and flexible.
Some common use cases for decorators include logging, caching, and access control. Decorators can also be used to add functionality to class methods, such as logging execution time or applying access control.
It's important to note that decorators can make your code more difficult to understand if used excessively or in a confusing way, so it's always good to use them judiciously and make sure they are well-documented.
In this post, we've covered the basics of decorators in Python and provided examples of how to use them in your own projects.