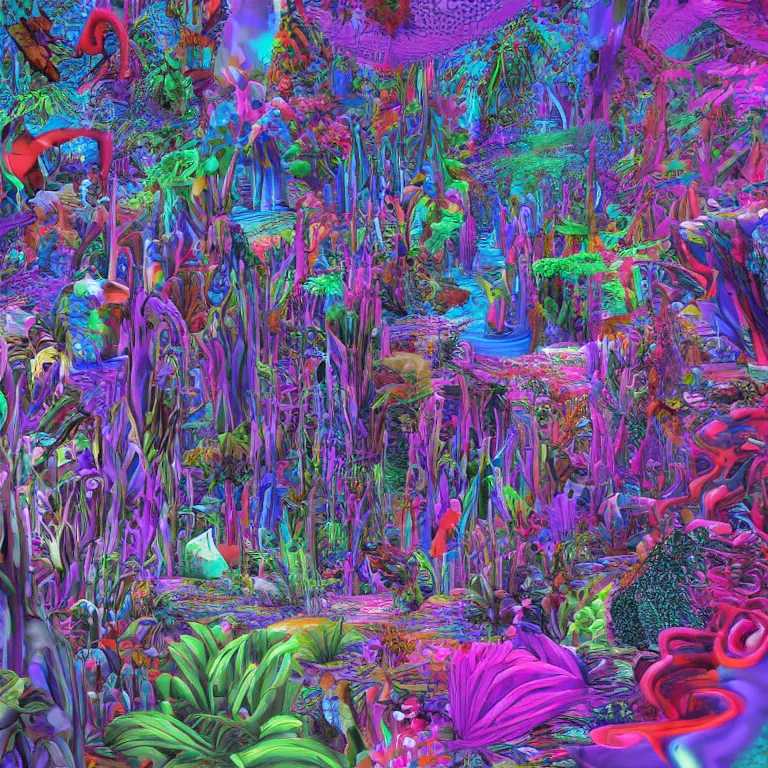
Feb 3rd, 2023
NumPy is a powerful, open-source Python library for numerical computation and data manipulation. It is built on the foundation of Python's array object and provides a wide range of mathematical functions and tools for working with arrays and matrices of numerical data.
Here are some of the most commonly used methods in the NumPy
library:
np.array(data, dtype=None, copy=True, order='K', subok=False, ndmin=0)
: This function creates an array from the given input data. Thedata
parameter can be a list, tuple, or array-like object, and thedtype
parameter specifies the data type of the resulting array. Thecopy
parameter, if set toTrue
, creates a copy of the input data, and theorder
parameter specifies the memory layout of the resulting array.
import numpy as np a = np.array([1, 2, 3, 4])
np.zeros(shape, dtype=float, order='C')
: This function returns a new array of given shape and type, filled with zeros. Theshape
parameter specifies the shape of the array and thedtype
parameter specifies the data type of the array.
np.zeros((3,3))
np.ones(shape, dtype=None, order='C')
: This function returns a new array of given shape and type, filled with ones. Theshape
parameter specifies the shape of the array and thedtype
parameter specifies the data type of the array.
np.ones((2,2))
np.arange(start, stop, step, dtype)
: This function returns an array with evenly spaced values within a given range. Thestart
parameter specifies the start of the range, thestop
parameter specifies the end of the range, and thestep
parameter specifies the spacing between the values. Thedtype
parameter specifies the data type of the array.
np.arange(1, 10, 2)
np.linspace(start, stop, num, endpoint, retstep, dtype)
: This function returns an array with evenly spaced values within a given range. Thestart
parameter specifies the start of the range, thestop
parameter specifies the end of the range, and thenum
parameter specifies the number of elements in the resulting array. Theendpoint
parameter, if set toTrue
, includes the end value in the range. Theretstep
parameter, if set toTrue
, returns the step value used in the range. Thedtype
parameter specifies the data type of the array.
np.linspace(1, 10, 6)
np.random.random(size=None)
: This function returns random floats in the half-open interval [0.0, 1.0). Thesize
parameter specifies the shape of the array.
np.random.random((3,3))
np.min(a, axis=None, out=None, keepdims=False)
: This function returns the minimum of an array or minimum along an axis. Thea
parameter specifies the input array, theaxis
parameter specifies the axis along which the minimum value is calculated and theout
parameter specifies the output and thekeepdims
parameter, if set toTrue
, keeps the dimensions of the input array.
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) np.min(a, axis=0)
np.max(a, axis=None, out=None, keepdims=False)
: This function returns the maximum of an array or maximum along an axis. Thea
parameter specifies the input array, theaxis
parameter specifies the axis along which the maximum value is calculated and theout
parameter specifies the output and thekeepdims
parameter, if set toTrue
, keeps the dimensions of the input array.
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) np.max(a, axis=0)
np.mean(a, axis=None, dtype=None, out=None, keepdims=False)
: This function returns the mean of an array or mean along an axis. Thea
parameter specifies the input array, theaxis
parameter specifies the axis along which the mean value is calculated, thedtype
parameter specifies the data type of the output, theout
parameter specifies the output and thekeepdims
parameter, if set toTrue
, keeps the dimensions of the input array.
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) np.mean(a, axis=0)
np.median(a, axis=None, out=None, overwrite_input=False, keepdims=False)
: This function returns the median of an array or median along an axis. Thea
parameter specifies the input array, theaxis
parameter specifies the axis along which the median value is calculated, theout
parameter specifies the output, theoverwrite_input
parameter, if set toTrue
, allows the input array to be modified and thekeepdims
parameter, if set toTrue
, keeps the dimensions of the input array.
a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) np.median(a, axis=0)
These are just a few examples of the many methods and capabilities of the NumPy library. NumPy is a fundamental library for scientific computing and data analysis in python, it provides the array data structure that is at the core of the pandas and many other popular data manipulation libraries. I hope this gives you a good introduction to using NumPy for numerical computation and data manipulation. Let me know if you have any questions or need more examples.