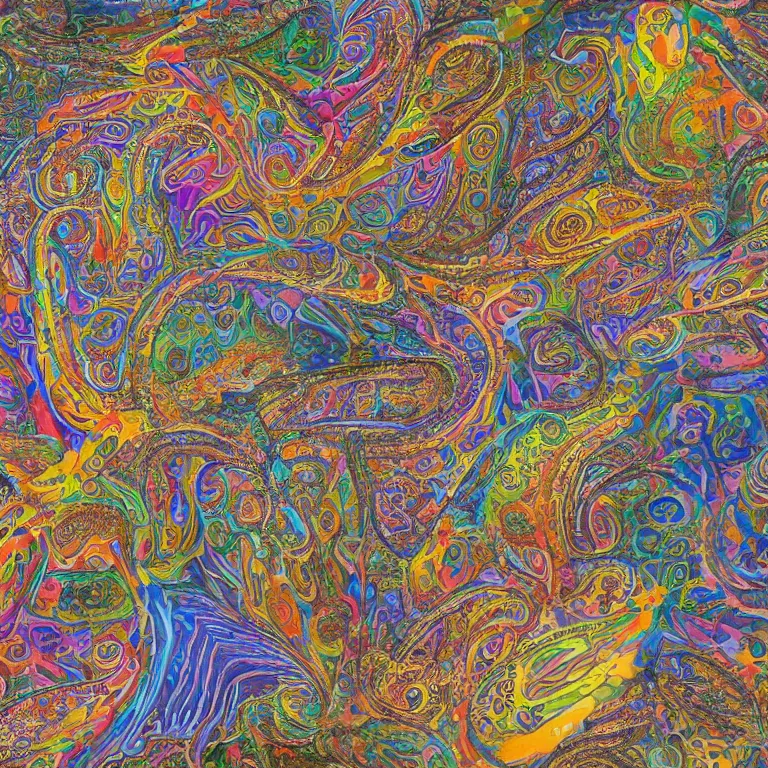
Feb 2nd, 2023
Pandas is a powerful, open-source Python library for data manipulation and analysis. It is built on top of the popular data manipulation library NumPy and provides easy-to-use data structures and data analysis tools for handling and manipulating numerical tables and time series data.
Pandas is widely used in professional careers, particularly in the field of data analysis and data science. It offers a wide range of functionalities that are essential in data preparation and cleaning, data manipulation, data visualization and statistical modeling. With pandas, you can easily handle large and complex datasets, perform various data transformations, and create intuitive data visualizations.
Pandas is especially useful for data cleaning and preprocessing, which is an important step in data analysis. It allows for easy handling of missing data, duplicate values, and data formatting issues. Data can be transformed and reshaped using various methods such as pivot tables, merging and joining, and filtering and subsetting.
Pandas is also an excellent tool for data exploration and visualization. It provides various functionalities for creating different types of plots, such as line plots, scatter plots, bar plots, and histograms. These plots can be customized with various styling options and can be easily exported to different file formats.
Pandas is widely used in various professional fields such as finance, marketing, and social sciences. It is used to analyze financial data, such as stock prices and financial statements, to identify patterns and trends. In marketing, pandas is used to analyze customer data and create customer segments, and in social sciences, it is used to analyze survey data and create statistical models. Pandas is also commonly used in data science and machine learning, where it is used to prepare data for modeling, feature engineering and data visualization.
Here are some of the most commonly used methods in the pandas
library:
pd.read_csv(filepath)
: This method reads a CSV file and converts it into a DataFrame, which is the primary data structure used in Pandas.
import pandas as pd data = pd.read_csv('data.csv')
DataFrame.head()
: This method returns the first n rows of a DataFrame, where n is 5 by default. It's a useful method for quickly previewing the data.
data.head()
DataFrame.info()
: This method provides a concise summary of a DataFrame, including the number of rows, number of columns, column data types, and memory usage.
data.info()
DataFrame.describe()
: This method provides summary statistics of the numerical columns in a DataFrame, including the count, mean, standard deviation, minimum, and maximum.
data.describe()
DataFrame.columns
: This attribute returns the column labels of a DataFrame.
data.columns
DataFrame.groupby(by)
: This method groups the rows of a DataFrame by the values in one or more columns, and applies a function to the grouped data.
data.groupby('column_name').mean()
DataFrame.sort_values(by, axis, ascending, inplace)
: This method sorts the rows of a DataFrame by the values in one or more columns. The by parameter specifies the column(s) to sort by, theaxis
parameter specifies 0 or 'index' for sorting rows and 1 or 'columns' for sorting columns, and theascending
parameter specifies whether to sort in ascending or descending order.
data.sort_values(by='column_name', ascending=False)
DataFrame.to_csv(filepath)
: This method writes a DataFrame to a CSV file.
data.to_csv('data_new.csv')
These are just a few examples of the many methods and capabilities of the Pandas library. I hope this gives you a good introduction to using Pandas for data manipulation and analysis. Let me know if you have any questions or need more examples.